Angular Injectable decorator - Expected 0 arguments but got 1
Solution 1
This is a new feature in Angular 6.
@Injectable({
providedIn: 'root'
})
You have two options:
-
Refer to the Angular 5 documentation. (As @r-richards notes - change the version of the docs to v5 in the dropdown at the bottom of the left nav.)
-
Upgrade your project to Angular 6 to fix the issue
Follow the official Angular upgrade guide.
You'll fill out a short form selecting which version of Angular you are on and which version you want to upgrade to. It then shows you the list of necessary steps to take to perform the upgrade. You should follow this guide for all upgrades.
Here are some notes I took while upgrading from Angular 5 to Angular 6.
Your mileage may vary depending on your configuration. I linked to a couple articles below that helped me.
Update npm (Node Package Manager)
npm install npm@latest -g
Upgarde node
For Windows and mac users, the easiest method is with the official Node installer. Or see the article below for linux/unix.
https://nodejs.org/en/download/
Upgarde Angular CLI globally and locally
npm install -g @angular/cli
npm install @angular/cli
Update package.json
ng update @angular/cli
Update @Angular/Core
ng update @angular/core
Update Angular Material if you're using it
ng update @angular/material
Check for other packages that may need to be upgraded
npm outdated
Update all outdated packages
npm update
Or to upgrade a specific package
npm update <package name>
You may need to convert the angular-cli.json file to angular.json
ng update @angular/cli --migrate-only --from=1.7.4
And as @Sharondio notes, it never hurts to restart your IDE, and possibly your entire computer at this point if you're seeing weirdisms.
References:
Upgrading Node
http://www.hostingadvice.com/how-to/update-node-js-latest-version/
Upgrading Angular
https://vitalflux.com/upgrade-angular-5-app-angular-6/
Other Troubleshooting Steps
https://stackoverflow.com/a/49811824/151325
Solution 2
I also had this problem, and I have resolved it including the name of the class service in "Providers" in app.module.ts.
like this:
@NgModule({
declarations:[
],
imports:[
CommonModule,
HttpClientModule
],
exports:[
],
providers: [
PostListService
]
})
Solution 3
The simplest solution to apply in Angular tutorial is:
1) Remove the unexpected argument in @Injectable decorator (due to your Angular version):
Do not use:
@Injectable({
providedIn: 'root',
})
export class HeroService {
But use:
@Injectable()
export class HeroService {
2) Add HeroService and MessageService to app.module.ts to avoid a No provider for HeroService! execution error due to dependecy injection:
app.module.ts @NgModule metadata:
providers: [
HeroService,
MessageService
],
Solution 4
for angular 5 you need to NOT set the parameter and leave like
@Injectable(/* without any parameter here for angular 5 */)
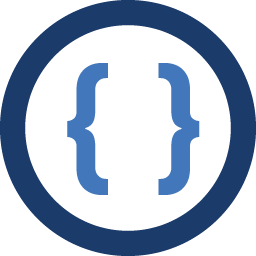
Admin
Updated on October 27, 2020Comments
-
Admin over 3 years
I am just getting started with the base tutorial for Angular but I face a small compilation issue.
I am following the tutorial on Angular's website. I am stuck with the dependency injection part because it doesn't want to compile.
In the tutorial they ask to create a service with :
ng generate service hero
In this generated service you have a decorator like this :
@Injectable()
Then they ask to add a parameter to that decorator like this :
@Injectable({ providedIn: 'root' })
When I try to do so I have a TypeScript error telling me :
error TS2554: Expected 0 arguments, but got 1. The versions I use are those ones :
Angular CLI: 1.7.4 Node: 9.5.0 OS: win32 x64 Angular: 5.2.10 ... animations, common, compiler, compiler-cli, core, forms ... http, language-service, platform-browser ... platform-browser-dynamic, router @angular/cli: 1.7.4 @angular-devkit/build-optimizer: 0.3.2 @angular-devkit/core: 0.3.2 @angular-devkit/schematics: 0.3.2 @ngtools/json-schema: 1.2.0 @ngtools/webpack: 1.10.2 @schematics/angular: 0.3.2 @schematics/package-update: 0.3.2 typescript: 2.5.3 webpack: 3.11.0
Any idea why it doesn't accept the parameter ?
Thanks in advance !
-
Admin almost 6 yearsYou have the links to node and angular flipped. You may want to edit this.
-
Francisco d'Anconia almost 6 yearsFixed. Good catch.
-
Sharondio almost 6 yearsAnd the important part (that only took me 45 minutes or so to figure out)...restart your IDE so it recognizes the right TS Linting.
-
Andrew Gray over 5 yearsAs of Angular 6, this is not the recommended way to register a Service with your modules; the
@Injectable({ providedIn: '???' })
decorator is what should be done, in general. If you need to use an earlier version, what you say in your answer is how you should do it.