Angular tests failing with Failed to execute 'send' on 'XMLHttpRequest'
Solution 1
This is a problem of the new Angular Cli. Run your test with --sourcemaps=false
and you will get the right error messages.
See details here: https://github.com/angular/angular-cli/issues/7296
EDIT:
Shorthand for this is:
ng test -sm=false
As of angular 6 the command is:
ng test --source-map=false
Solution 2
I had the same issue using angualar cli 6, I have used this tag to get the right error message :
ng test --source-map=false
Maybe it will help someone :) .
Solution 3
For my case there was a mock data problem and in case of Array
, I was returning string
from the mock.
someApi = fixture.debugElement.injector.get(SomeApi);
spyOn(someApi, 'someMethod')
.and.returnValue(Observable.of('this is not a string but array'));
The error message is really distracting and was not telling the actual error. Running
ng test --source=false
pointed the correct error and line, and helped me to fix it quickly.
Many time it happens when you mock data is incomplete or incorrect.
Solution 4
You can either set input() property to default value in component.ts
@Input() tableColumns: Array<any> = [];
@Input() pageObj: any = '';
OR
Modify your component.spec.ts file in following way,
beforeEach(() => {
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
component.tableColumns = [];
component.pageObj = '';
fixture.detectChanges();
});
Solution 5
As suggested above here: https://stackoverflow.com/a/45570571/7085047 my problem was in my ngOnInit
. I was calling a mock swagger-generated REST controller proxy. It was returning null, and I was subscribing to that null, which doesn't work...
The error came back:
Failed to load ng:///DynamicTestModule/MockNodeDashboardComponent_Host.ngfactory.js: Cross origin requests are only supported for protocol schemes: http, data, chrome, chrome-extension, https.
I fixed the issue using ts-mockito: https://github.com/NagRock/ts-mockito
I added code to create a mock instance like this:
import { mock, instance, when } from 'ts-mockito';
import { Observable } from 'rxjs/Observable';
import { Observer } from 'rxjs/Observer';
import { MockScenario } from './vcmts-api-client/model/MockScenario';
const MockVcmtsnodemockresourceApi: VcmtsnodemockresourceApi = mock(VcmtsnodemockresourceApi);
const obs = Observable.create((observer: Observer<MockScenario[]>) => {
observer.next(new Array<MockScenario>());
observer.complete();
});
when(MockVcmtsnodemockresourceApi.getMockScenariosUsingGET()).thenReturn(obs);
const instanceMockVcmtsnodemockresourceApi: VcmtsnodemockresourceApi = instance(MockVcmtsnodemockresourceApi);
And then added the instance to the test's providers array like this:
beforeEach(async(() => {
TestBed.configureTestingModule({
...
providers: [
...
{ provide: VcmtsnodemockresourceApi, useValue: instanceMockVcmtsnodemockresourceApi },
...
]
}).compileComponents();
}));
Related videos on Youtube
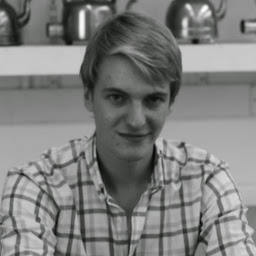
George Edwards
Updated on July 08, 2022Comments
-
George Edwards almost 2 years
I am trying to test my angular 4.1.0 component -
export class CellComponent implements OnInit { lines: Observable<Array<ILine>>; @Input() dep: string; @Input() embedded: boolean; @Input() dashboard: boolean; constructor( public dataService: CellService, private route: ActivatedRoute, private router: Router, private store: Store<AppStore>) { } }
However, a simple "should create" test throws this cryptic error...
NetworkError: Failed to execute 'send' on 'XMLHttpRequest': Failed to load 'ng:///DynamicTestModule/module.ngfactory.js'.
so I found this question, which suggests that the issue is the component has
@Input)_
params which aren't set, however, if I modify my test like so:it('should create', inject([CellComponent], (cmp: CellComponent) => { cmp.dep = ''; cmp.embedded = false; cmp.dashboard = false; expect(cmp).toBeTruthy(); }));
then I still get the same issue, similarly, if I remove the
@Input()
annotations from the component, still no difference. How can I get these tests to pass?-
Aleksandr Petrovskij almost 7 yearsIn order to create component, you need to provide all dependencies. Can you show all your test setup? I will try to reproduce the problem on plnkr
-
Niles Tanner almost 7 yearsI had this same issue and found the same posts that you did. I was able to find a solution. I ended up posting on the other question but you can take a look here: stackoverflow.com/a/45419372/6739517 Hope it helps!
-
titusfx over 6 years
-
-
Anton Balaniuc over 6 yearsIf you have a new question, please ask it by clicking the Ask Question button. Include a link to this question if it helps provide context. - From Review
-
Alan Smith over 6 yearsYou absolute hero. I was banging my head against the wall in frustration with the lack of info from Angular unit test error messages, until I found this. Much obliged.
-
Datum Geek over 6 yearsseemed like my symptom was the same as the OP, so i thought folks might find useful the fix that worked for me...
-
user1806692 about 6 yearsThis answer really saved my day! I was close to giving up on development in general after spending whole day and night trying to fix this just so I could stop being the person failing the build
-
peng about 6 yearsToday i came across this error message: HeadlessChrome 65.0.3325 (Mac OS X 10.13.4) ERROR { "message": "Script error.\nat :0:0", "str": "Script error.\nat :0:0" } And removing --sourcemap=false shows more information.
-
danday74 almost 6 yearsng test --source-map=false ... works in Angular CLI 6
-
Brad Richardson almost 6 years@danday74 FTW! After writing complicated test files, getting hung up on this is brutal.
-
Amir Khademi almost 6 yearsdoesn't work in angular cli 6.0.8 instead, use ng test --source-map=false
-
philip yoo over 3 yearsng test --sourceMap=false ... works in angular cli v9.1.12