Angular: Unit test that a component's output is not emitted
13,439
Solution 1
You can use a spy like this:
describe('ExampleComponent', () => {
it('should not output duplicate values', () => {
const component = new ExampleComponent();
spyOn(component.output, 'emit');
component.value = 1;
component.onValueChange(1);
expect(component.output.emit).not.toHaveBeenCalled();
});
});
Solution 2
That's pretty much how you do it. A variation is:
describe('ExampleComponent', () => {
it('should not output duplicate values', () => {
const component = new ExampleComponent();
let numEvents = 0;
component.value = 1;
component.output.subscribe(value => ++numEvents);
component.onValueChange(1);
expect(numEvents).toEqual(0);
});
});
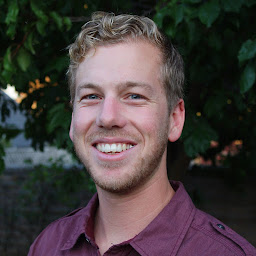
Author by
Rich McCluskey
Updated on June 06, 2022Comments
-
Rich McCluskey almost 2 years
Let's say I have a component like the following:
@Component({ selector: 'example', template: ` ` }) export class ExampleComponent { value: any; @Output() output: EventEmitter<any> = new EventEmitter(); onValueChange(newValue: any) { if (newValue !== this.value) { this.value = newValue; this.output.emit(newValue); } } }
I've written a test like the one below. I want to test that if
onValueChange
is called with the same value asvalue
, the component will not output the duplicate value. Is there a best practice for unit testing that an observable subscription is never called? While what I did technically works, it feels a little hacky.describe('ExampleComponent', () => { it('should not output duplicate values', () => { const component = new ExampleComponent(); component.value = 1; component.output.subscribe(value => { // if the output is not triggered then we'll never reach this // point and the test will pass expect(true).toEqual(false); }); component.onValueChange(1); }); });
-
Tsvetan Ganev over 2 yearsAnd if you're using Jest:
jest.spyOn()
.