Angular2 - Generate pdf from HTML using jspdf
Solution 1
What I found worked was adding:
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/0.4.1/html2canvas.js"></script>
to the index.html file (it could presumably be elsewhere).
I then used:
const elementToPrint = document.getElementById('foo'); //The html element to become a pdf
const pdf = new jsPDF('p', 'pt', 'a4');
pdf.addHTML(elementToPrint, () => {
doc.save('web.pdf');
});
Which no longer uses html2canvas in the code.
You can then remove the following import:
import * as html2canvas from 'html2canvas';
Solution 2
In case someone prefer not to use cdn scripts & would like to use a more (angular) way, this worked for me in Angular 6:
Using this way will give you better support & autocomplete in the editor & will help you avoid depending on cdn scripts (if you wanna avoid them, like me)
Based on the excellent answer here & since it was hard for me to find that answer, I am re-sharing what was stated in it & helped me use jsPDF in Angular 6 (all credit goes to the original author of this answer)
You should run these cmds:
npm install jspdf --save
typings install dt~jspdf --global --save
npm install @types/jspdf --save
Add following in angular-cli.json:
"scripts": [ "../node_modules/jspdf/dist/jspdf.min.js" ]
html:
<button (click)="download()">download </button>
component ts:
import { Component, OnInit, Inject } from '@angular/core';
import * as jsPDF from 'jspdf'
@Component({
...
providers: [
{ provide: 'Window', useValue: window }
]
})
export class GenratePdfComponent implements OnInit {
constructor(
@Inject('Window') private window: Window,
) { }
download() {
var doc = new jsPDF();
doc.text(20, 20, 'Hello world!');
doc.text(20, 30, 'This is client-side Javascript, pumping out a PDF.');
doc.addPage();
doc.text(20, 20, 'Do you like that?');
// Save the PDF
doc.save('Test.pdf');
}
}
Solution 3
Anyone still trying to convert an Html div to a pdf can opt to use html2pdf, with a couple of lines you can do everything with ease.
var element = document.getElementById('element-to-print');
html2pdf(element);
Solution 4
Try it like this:
GeneratePDF () {
html2canvas(this.element.nativeElement, <Html2Canvas.Html2CanvasOptions>{
onrendered: function(canvas: HTMLCanvasElement) {
var pdf = new jsPDF('p','pt','a4');
var img = canvas.toDataURL("image/png");
pdf.addImage(img, 'PNG', 10, 10, 580, 300);
pdf.save('web.pdf');
}
});
}
Solution 5
Use this way stackblitz example
import {jsPDF} from 'jspdf';
@ViewChild('content', {static: false}) content: ElementRef;
public downloadPDF() {
const doc = new jsPDF();
const specialElementHandlers = {
'#editor': function (element, renderer) {
return true;
}
};
const content = this.content.nativeElement;
doc.fromHTML(content.innerHTML, 15, 15, {
width: 190,
'elementHandlers': specialElementHandlers
});
doc.save('fileName.pdf');
}
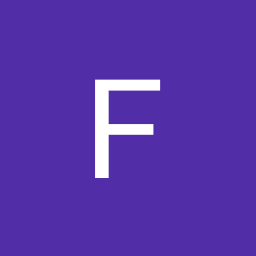
FlorisdG
Front-end web-developer by day, game-developer by night.
Updated on July 05, 2022Comments
-
FlorisdG almost 2 years
For a project I'm working on I need to be able to generate a PDF of the page the user is currently on, for which I'll use
jspdf
. Since I have aHTML
I need to generate a PDF with, I'll need theaddHTML()
function. There are a lot of topic about this, sayingYou'll either need to use
html2canvas
orrasterizehtml
.I've chosen to use
html2canvas
. This is what my code looks like at the moment:import { Injectable, ElementRef, ViewChild } from '@angular/core'; import * as jsPDF from 'jspdf'; import * as d3 from 'd3'; import * as html2canvas from 'html2canvas'; @Injectable () export class pdfGeneratorService { @ViewChild('to-pdf') element: ElementRef; GeneratePDF () { html2canvas(this.element.nativeElement, <Html2Canvas.Html2CanvasOptions>{ onrendered: function(canvas: HTMLCanvasElement) { var pdf = new jsPDF('p','pt','a4'); pdf.addHTML(canvas, function() { pdf.save('web.pdf'); }); } }); } }
When this function is called, I get a console error:
EXCEPTION: Error in ./AppComponent class AppComponent - inline template:3:4 caused by: You need either https://github.com/niklasvh/html2canvas or https://github.com/cburgmer/rasterizeHTML.js
Why exactly is this? I give a
canvas
as parameter and it still says I need to usehtml2canvas
. -
Tim Schimandle about 7 yearsWould be nice if the import statement worked. But This should be selected as the answer for now.
-
Vignesh over 6 years@Greg without importing the script in index.html. Can we able to do load html2canvas.js
-
Pratap A.K over 6 yearswhere are you using html2canvas in your code apart from importing? I'm getting error You need either github.com/niklasvh/html2canvas or github.com/cburgmer/rasterizeHTML.js Error: You need either github.com/niklasvh/html2canvas or github.com/cburgmer/rasterizeHTML.js at Object.e.API.addHTML
-
balazs630 over 6 yearsThanks, this solution worked for me in an Angular 5 project! Adding the script in index.html is crucial, if you only add it in angular.cli.json, it will not work somehow. The scipt needs to be in index.html.This is the newest version right now: 0.5.0-beta4
-
Vignesh over 6 yearsI tried your suggestion it worked fine after updating the packages to latest version >"html2canvas:1.0.0-alpha.9", "jspdf": "^1.3.5", "jquery": "^3.3.1". Generating of pdf from HTML using jspdf is not working.
-
Sadiq Ali about 6 yearsCan you let me know the reason for the down vote? html2pdf uses jspdf internally and it makes things a lot easier. Someone might find it useful as this question is what most people stumble upon.
-
akcasoy about 4 years@Vignesh "npm i html2canvas", and add the file path ("node_modules/html2canvas/dist/html2canvas.js") in your angular.json scripts array.