angular2 http.post() to local json file
Solution 1
Unfortunately, you can not PUT or POST directly to a file on your local filesystem using client side (browser) JavaScript.
Consider building a simple server to handle a POST request. If you are most comfortable with JavaScript, try Express with Node.js
// server.js
'use strict'
const bodyParser = require('body-parser');
const express = require('express');
const fs = require('fs');
const app = express()
app.use(bodyParser.json());
app.use(function(req, res, next) {
res.header('Access-Control-Allow-Origin', 'http://localhost:8000');
res.header('Access-Control-Allow-Headers', 'Origin, X-Requested-With, Content-Type, Accept');
res.header('Access-Control-Allow-Methods', 'POST');
next();
});
app.post('/', (req, res) => {
console.log('Received request');
fs.writeFile('json.json', JSON.stringify(req.body), (err) => {
if (err) throw err;
console.log('File written to JSON.json');
res.send('File written to JSON.json')
})
});
app.listen(3000, ()=>{
console.log('Listening on port 3000. Post a file to http://localhost:3000 to save to /JSON.json');
});
Solution 2
You cannot write to files from Browser. Period. Even if such action is possible it will be stored on User side, not your server. If your task is indeed to write something on user end, please refer to localStorage documentation (or couple other API implementations). But if you are going to user that file for some purpose outside of your browser, it will be non-trivial task.
If you want to save file on server, then you need to hande it on back end.
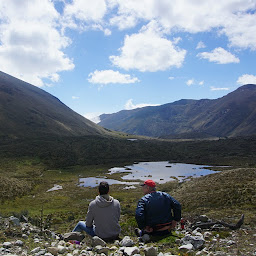
Guy K
Updated on June 18, 2022Comments
-
Guy K almost 2 years
little help?
I am trying to write pure JSON to a file inside my project, my project tree looks like this:
src ->app -->components --->people.component.ts --->(other irrelevant components) -->services --->people.service.ts -->data --->JSON.json ->(other irrelevant src files)
The code in my
people.component.ts
is just used to call and subscribe to a function inside mypeople.service.ts
, passing thenewPerson
property which is data-binded from the DOM using angular2 magic; this is the function insidename.service.ts
:public addPerson(newPerson) { let headers = new Headers(); headers.append('Content-Type', 'application/json'); return this.http.post('app/data/PEOPLE.json', newPerson, { header: headers }) .map(res => res.json()) }
My objective is to write (if necessary replace) the
newPerson
property (or the entire file) insidePEOPLE.json
. of course, the currentaddPerson()
function returns an error.I've also tried the
put
method, it errors slightly differently, but i haven't found solutions to either method.I know categorically it's not an error with the format/type of data i'm trying to put in
PEOPLE.json
file.