Angular2 service testing : inject a dependency with beforeEach
24,687
Is it possible to use a beforeEach to inject the dependency before every test ?
Sure you could.
let service;
beforeEach(inject([Service], (svc) => {
service = svc;
}))
Though you could also just get the service from the TestBed
, which is also an injector
let service;
beforeEach(() => {
TestBed.configureTestingModule({
...
})
service = TestBed.get(Service);
})
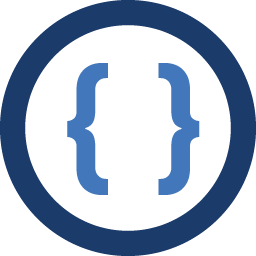
Author by
Admin
Updated on April 21, 2020Comments
-
Admin about 4 years
I am testing services with an
Http
dependency. Every test looks like this :import { TestBed, async, inject } from '@angular/core/testing'; import { ValidationService } from './validation.service'; import { HttpModule, Http, Response, ResponseOptions, RequestOptions, Headers, XHRBackend } from '@angular/http'; import { MockBackend, MockConnection } from '@angular/http/testing'; describe('DashboardService', () => { beforeEach(() => { TestBed.configureTestingModule({ imports: [HttpModule], providers: [ ValidationService, { provide: XHRBackend, useClass: MockBackend } ] }); }); it('should ...', inject([ValidationService, XHRBackend], (service: ValidationService, mockBackEnd: MockBackend) => { mockBackEnd.connections.subscribe((connection: MockConnection) => { connection.mockRespond(new Response(new ResponseOptions({ body: JSON.stringify('content') }))); }); })); // assertions ... });
As you can see, I need to inject the BackEnd mock at every it.
Is it possible to use a
beforeEach
to inject the dependency before every test ?