AngularJS - Create dynamic properties to an object/model from json
11,222
You can use angular.forEach
and the bracket notation for setting (and getting) object properties in Javascript
var myObject = {}
DynamicObjSvc.get().success(
function(data) {
angular.forEach(data, function(value, key) {
myObject[key] = value;
});
}
);
See also Working with Objects from MDN
EDIT
I see now that your data
is really an array of objects, not just a single object, so yes, the code above could lead you astray.
In any case, the method of setting an object's properties dynamically using the bracket notation is sound; the loop could be reworked to handle your data
array as such:
//we have an array of objects now
var myObjects = [];
DynamicObjSvc.get().success(
function(data) {
//for each object in the data array
for(var i = 0; i < data.length; i++) {
//create and populate a new object for the ith data element
var newObject = {};
angular.forEach(data[i], function(value, key) {
newObject[key] = value;
});
//and add it to the overall collection
myObjects.push(newObject);
}
}
);
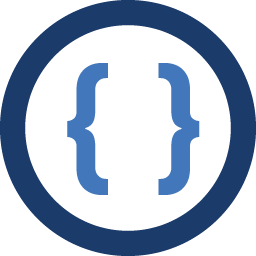
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
OK, we all know this works:
vm.myObject = { required : "This field requires data", ..... }
But how can I create that same object dynamically when the property 'keys' and 'values' come from a json file, eg:
json:
[ { "key" :"required", "value": "This field requires data"}, ..... ]
service:
var myObject = {} DynamicObjSvc.get() .success(function(data){ data.forEach(function(item){ // pass each key as an object property // and pass its respective value ????????? }) .....
UPDATE:
Kavemen was mostly correct, this turned out to be the solution:
var myObject = {}; DynamicObjSvc.all() .success(function(data){ angular.forEach(data, function(msg) { myObject[msg.key] = msg.value; <-- his answer was incorrect here }); $fgConfigProviderRef.validation.message(myObject); }) .error(function(err){ console.log(err.message); })