AngularJS Route Controller Not Reloading
Solution 1
When you navigate to the same page, the browser ignores it.
Angular.js has nothing to do with the default behavior of <a href="">
.
What you can do, is to create a custom directive which:
- use
$route.reload()
to reload the current view (route). - use
$location.path(newPath)
for navigating to other views.
I made an example:
app.directive('xref',function($route, $location){
return {
link: function(scope, elm,attr){
elm.on('click',function(){
if ( $location.path() === attr.xref ) {
$route.reload();
} else {
scope.$apply(function(){
$location.path(attr.xref);
});
}
});
}
};
});
In your view just create:
<a xref="/">Home</a>
<a xref="/links">Links</a>
Solution 2
You could check if the current route is the same as the "destination" route and call $route
's reload()
method:
reload()
Causes $route service to reload the current route even if $location hasn't changed.
As a result of that, ngView creates new scope, reinstantiates the controller.
E.g.:
In HTML:
<a href="" ng-click="toResults()">Results</a>
In controller:
// ...first have `$location` and `$route` injected...
$scope.toResults = function () {
var dstPath = '/results';
if ($location.path() === dstPath) {
$route.reload() ;
} else {
$location.path(dstPath);
}
}
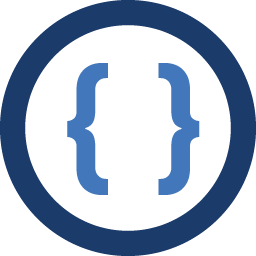
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I have a very simple AngularJS app that has two routes in it:
#/search #/results
When I navigate from one route to another everything works as I'd expected. Any resources that are needed are fetched and the content is displayed perfectly.
The problem is when I navigate from one route to the same route (I.e., #/results to #/results) absolutely nothing happens. I understand from the browser's perspective nothing has happened but I'd really like AngularJS to reload the content.
This must be very easy but I'm drawing blanks on this. Can someone please shed some light on this for me?
Thanks,
Jon
-
Admin over 10 yearsThank you very much. That's exactly what I'll do. Should be easy.
-
Admin over 10 yearsI ended up using this simple directive instead. So awesome. Thanks
-
Anuj over 7 yearsi have injected $route in my Logout controller but i am getting "[$injector:unpr] Unknown provider: $routeProvider <- $route <- LogoutCtrl" issue.Help me out
-
gkalpak over 7 yearsInclude the
ngRoute
module as a dependency of your app. Everything you need is in thw docs.