Anonymous/inline interface implementation in TypeScript
OK, I finally discovered the problem to question 2 - I was using the fat arrow =>
to declare the object's method here:
doThatThing(<Doable>{
private message: 'ahoy-hoy!',
do: () => { // using fat arrow: global scope replaces new object's scope
alert(this.message);
}
});
...which "sucked" the global scope into the method. The problem is fixed using the longer syntax, like so:
doThatThing(<Doable>{
private message: 'ahoy-hoy!',
do: function() { // using "regular" anonymous function syntax, "this" meaning is preserved
alert(this.message);
}
});
So in summary:
- unanswered;
- There was a typo in my code, and I should have been using "function()" instead of "=>"; and,
- Type-asserting the object with the interface removes the compiler error.
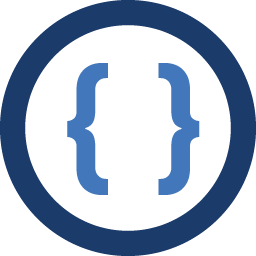
Admin
Updated on September 18, 2020Comments
-
Admin over 3 years
I've just started with TypeScript and I'm trying to understand why the following inline object definition isn't considered valid. I have a collection of objects - their type is irrelevant (to me), but they implement the interface so that when I iterate through them I know that the interface methods will be present in each object in the collection.
I came across a "compiler" error when I tried to create an object with private information required to implement the required method:
interface Doable { do(); } function doThatThing (doableThing: Doable) { doableThing.do(); } doThatThing({ private message: 'ahoy-hoy!', // compiler error here do: () => { alert(this.message); } });
The compiler error message is "Argument of type
'{ message: string, do: () => void; }'
is not assignable to type Doable. Object literal must specify known properties, and 'message' does not exist in type Doable". Note that the same message is given if I define the object outside of the function call, i.e.var thing: Doable; thing = { private message: 'ahoy-hoy!', // error here do: () => { alert(this.message); } }; doThatThing(thing);
The same error occurs if I add "unexpected" methods as well:
doThatThing({ do: () => { alert("ahoy hoy"); }, doSecretly: () => { // compiler error here now alert("hi there"); } });
I looked at the JavaScript and discovered that
this
within the inline object definition was being scoped to the global object:var _this = this; // wait, no, why!? function doThatThing(doableThing) { doableThing.do(); } doThatThing({ message: 'ahoy-hoy!', do: function () { alert(_this.message); // uses global } });
I tried searching for information on inline implementations of interfaces in TypeScript, but couldn't find anything speaking to this issue specifically.
I can confirm that the "fixed" compiled JS works as intended:
function doThatThing(doableThing) { doableThing.do(); } doThatThing({ message: 'ahoy-hoy!', do: function () { alert(this.message); } });
...and that makes sense to me, because (as far as I understand) this is implicitly calling the Object constructor, so
this
should be scoped to the new Object instance.It seems like the only solution is to declare each implementation as a class implementing the interface, but that feels really regressive/heavy-handed since I'm only going to have one instance of each class. If the only contract with the called function is implementing the interface, then why can't the object contain additional members?
Sorry, this turned out longer than I intended ...in summary, I'm asking:
- Why is that inline interface implementation ("anonymous class", as would be said in Java) considered invalid in TypeScript? Specifically, what does that compiler error mean, and what does it protect against?
- Why is the scope-reassignment to the global object generated in the "compiled" JavaScript?
- Assuming it's my error (e.g. that the compiler error is necessary for protecting against some undesirable condition), is the only solution really to explicitly declare a class in advance, like so?
interface Doable { do() : void; } class DoableThingA implements Doable { // would prefer to avoid this ... private message: string = 'ahoy-hoy'; do() { alert(this.message); } } class DoableThingB implements Doable { // ... as well as this, since there will be only one instance of each do() { document.getElementById("example").innerHTML = 'whatever'; } } function doThatThing (doableThing: Doable) { doableThing.do(); } var things: Array<Doable>; things = new Array<Doable>(); things.push(new DoableThingA()); things.push(new DoableThingB()); for (var i = 0; i < things.length; i++) { doThatThing(things[i]); }
P.S. The compiler error only appeared when I upgraded to TS 1.6 today, although the faulty scope bug in the compiled JS occurs in both 1.6 and 1.5.
Update: François Cardinaux provided a link to this answer, which recommends using a type assertion, but this only removes the compiler error and actually causes a logic error due to improper scope:
interface Doable { do(); } function doThatThing (doableThing: Doable) { doableThing.do(); } doThatThing(<Doable>{ // assert that this object is a Doable private message: 'ahoy-hoy!', // no more compiler error here do: () => { alert(this.message); } });
Looking at the compiled JS, this is incorrect:
var _this = this; // very wrong, and now hidden function doThatThing(doableThing) { doableThing.do(); } doThatThing({ message: 'ahoy-hoy!', do: function () { alert(_this.message); // s/b "this.message", which works in JS (try it) } });
-
Leon Adler about 8 yearsAs a sidenote for future readers: Inline functions can also be defined without the
function
keyword:{ message: 'ahoy-hoy!', do() { alert(this.message); }}
. This syntax is just a shorthand for a normal function and does not bind a lexicalthis
like arrow functions. -
Rycochet about 7 yearsShould have used
do=()=>{ ... }
instead - the assignment makes sure it's using theDoable
instance scope even if called from an event handler etc.