Api annotation's description is deprecated
Solution 1
I found two solutions for Spring Boot application:
1. Swagger 2 based:
Firstly, use the tags
method for specify the tags definitions in your Docket
bean:
@Configuration
@EnableSwagger2
public class Swagger2Config {
public static final String TAG_1 = "tag1";
@Bean
public Docket productApi() {
return new Docket(DocumentationType.SWAGGER_2).select()
.apis(RequestHandlerSelectors.basePackage("my.package")).build()
.tags(new Tag(TAG_1, "Tag 1 description."))
// Other tags here...
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder().title("My API").version("1.0.0").build();
}
}
After, in RestController
just add the @Api
annotation with one (or more) of the your tags:
@Api(tags = { SwaggerConfig.TAG_1 })
@RestController
@RequestMapping("tag1-domain")
public class Tag1RestController { ... }
2. Swagger 3 based (OpenAPI):
Similarly, use the addTagsItem
method for specify the tags definitions in your OpenAPI
bean:
@Configuration
public class OpenApiConfig {
public static final String TAG_1 = "tag1";
@Bean
public OpenAPI customOpenAPI() {
final Info info = new Info()
.title("My API")
.description("My API description.")
.version("1.0.0");
return new OpenAPI().components(new Components())
.addTagsItem(createTag(TAG_1, "Tag 1 description."))
// Other tags here...
.info(info);
}
private Tag createTag(String name, String description) {
final Tag tag = new Tag();
tag.setName(name);
tag.setDescription(description);
return tag;
}
}
Finally, in RestController
just add the @Tag
annotation:
@Tag(name = OpenApiConfig.TAG_1)
@RestController
@RequestMapping("tag1-domain")
public class Tag1RestController { ... }
Solution 2
This is the correct way to add description to your Swagger API documentation for Swagger v1.5:
@Api(tags = {"Swagger Resource"})
@SwaggerDefinition(tags = {
@Tag(name = "Swagger Resource", description = "Write description here")
})
public class ... {
}
Solution 3
The reason why it's deprecated is that previous Swagger versions (1.x) used the @Api
description annotation to group operations.
In the Swagger 2.0 specification, the notion of tags
was created and made a more flexible grouping mechanism. To be API compliant, the description
field was retained so upgrades would be easy, but the correct way to add a description is though the tags
attribute, which should reference a @Tag
annotation. The @Tag
allows you to provide a description and also external links, etc.
Solution 4
I tried above solutions but they didn't work for me.
To add a title and description to the documentation you create ApiInfo and Contact objects like in example below. Then you simply add apiInfo object to your Swagger Docket.
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
@EnableSwagger2
@Configuration
public class SwaggerConfig {
private Contact contact = new Contact("", "", "");
private ApiInfo apiInfo = new ApiInfo(
"Backoffice API documentation",
"This page documents Backoffice RESTful Web Service Endpoints",
"1.0",
"",
contact,
"",
"");
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo)
.select()
.apis(RequestHandlerSelectors.basePackage(
PaymentsController.class.getPackage().getName()
))
.paths(PathSelectors.ant("/api/v1/payments" + "/**"))
.build()
.useDefaultResponseMessages(false)
.globalOperationParameters(
newArrayList(new ParameterBuilder()
.name("x-authorization")
.description("X-Authorization")
.modelRef(new ModelRef("string"))
.parameterType("header")
.required(false)
.build()));
}
}
Above code produces a description like in a screenshot below.
Solution 5
I found that the following works by combining both the @Api
and @Tag
annotations building off of this answer.
The value within the tags field of the @Api
annotation needs to match the value within the name field of the @Tag
annotation.
@Api(tags = "Controller API")
@Tag(name = "Controller API", description = "This controller performs API operations")
public class ReportStatusConsumerController {
}
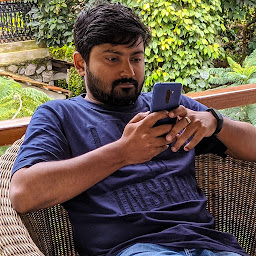
Soumitri Pattnaik
I am a Full-stack cloud developer having over to 8 years of professional experience and close to 12 years of experience in software development. In my day job, I predominantly work on Java, SpringBoot microservices, Python, Google Cloud services, and Kubernetes. Along with these technologies, I do a bit of Angular, Typescript on our frontend web app, I also manage a few small services written on Groovy and NodeJS / NestJS. While writing micro-services to run on Kubernetes, I hated typing long and error-prone K8S commands, so I wrote (using Electron) a little GUI (DevKube) for kubectl which made my and a bunch of people's day a bit easier. I am very passionate about making software that makes people's life easier. I not only enjoy writing code, but I also enjoy the grind of learning new things.
Updated on July 05, 2022Comments
-
Soumitri Pattnaik almost 2 years
In Swagger, the
@Api
annotation'sdescription
element is deprecated.Deprecated. Not used in 1.5.X, kept for legacy support.
Is there a newer way of providing the description?
-
Tristan over 5 yearsThis looks like a good way to do it, but what if you are reusing the tag on multiple APIs ? where would u put SwaggerDefinition ? I've tried to put it on my SwaggerConfig bean, but it was not taken into account. Only the programatically way worked for me.
-
Kick about 5 yearsDescription of Tag not coming on swagger UI
-
Roddy of the Frozen Peas about 5 years@Kick - It does if you use a new enough version of Swagger (eg. 2.9.x)
-
PAA about 5 years@falvojr - Perfect. However if there is any way if we can reduced font size?
-
LEVEL almost 5 years@zhuguowei, Did you found the way to use it in 2.9.2? Help me if you know how to use it in a 2.9.2 version.
-
zhuguowei almost 5 years@ShivarajaHN please see falvojr 's answer
-
Venky over 4 yearsGreat, This worked for me too. However, I am wondering if there is any way to add line breaks in the description. I tried putting \n and <br>
-
OzgurH over 4 yearsI've added your image - pending review... but it seems you may have answered another question here! The question is about
description
attribute of@Api
annotation - which was being rendered per class/tag/group of entry points, not the whole application's API as in your screenshot -
Anand Varkey Philips over 4 yearsThis helped for 2.9.2 version!
-
Zon over 4 yearsDon't you face the
new io.swagger.annotations.Tag
- is abstract, cannot be instantiated? Should bespringfox.documentation.service.Tag
. -
falvojr over 4 yearsYes, I'm using the
springfox.documentation.service.Tag
, following thetags
(builder method) signature. -
Dmitriy Popov over 4 yearsUnfortunately, this solution does not work, see github.com/swagger-api/swagger-core/issues/1476.
-
M. Justin over 3 yearsGitHub issue for this solution not working: github.com/swagger-api/swagger-core/issues/3737
-
Harihara_K about 3 yearsAn example would be much appreciated!
-
Sasha Bond about 3 yearsthe idea is correct, but not the implementation, for correct implementation see
1. Swagger 2 based:
from falvojr - set@Api(tags = {"User"})
- that User appears in Swagger page and in SwaggerConfig.java for new Docket() after build add.tags(new Tag("User", "some user actions");
-
Mariano Cali about 3 yearsso I have to add description inside Swagger2Config. So If I have 20 controllers I have to put all of them in the Swagger2Config. IMHO it would be better to have it inside the same controller