API Json response to C# Object with capital case properties first letter
Solution 1
By default, ASP.NET Core encodes all JSON properties names in camel case, to match JSON conventions (see the announcement of the change on GitHub).
If you want to keep the C# conventions, you need to change the default JSON serializer.
In your Startup.cs
, configure the MVC part like this (ASP.Net Core 3.0):
services
.AddMvc()
.AddNewtonsoftJson(options =>
{
// don't serialize with CamelCase (see https://github.com/aspnet/Announcements/issues/194)
jsonSettings.ContractResolver = new JsonContractResolver();
});
For ASP.NET Core 2.0 :
services
.AddMvc()
.AddJsonOptions(options =>
{
// don't serialize with CamelCase (see https://github.com/aspnet/Announcements/issues/194)
jsonSettings.ContractResolver = new DefaultContractResolver();
});
Solution 2
You have to change the default property naming policy on the json serialization options.
By default it's set to camel case but if you set it to null
, the property names are to remain unchanged (or remain as you wrote on your class).
Simply add this to your Startup.cs
:
services.AddControllers()
.AddJsonOptions(options =>
{
options.JsonSerializerOptions.PropertyNamingPolicy = null;
});
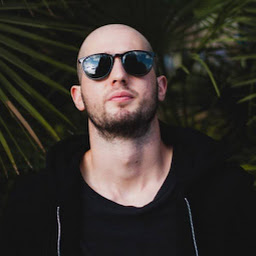
Alessandro Albi
C# [.NET Core, UWP, WPF, Xamarin] developer, I like to develop in the Microsoft environment but with an eye also on the outside
Updated on June 05, 2022Comments
-
Alessandro Albi almost 2 years
I've made an API where after an Entity Framework elaboration I send an object serialized in Json.
My Object:
public class Package { public int Items { get; set; } public string Code { get; set; } public string Description { get; set; } public double? Weight { get; set; } public string Size { get; set; } public string PackageType { get; set; } }
The problem start when after recieve it (Xamarin app) the Json have the first letter lowercase, but I want deserialize it in the exact same class and it can't because the class have properties in capitalcase (C# standard). Now I'm using a horrible 'helper' class that have the properties in lowercase for translating it.
Any idea how to handle this and send the Json directly with capital case first letter?
Edit
I use ASP.NET web API Core and Newtonsoft.Json
In Xamarin app I use System.Text.Json
-
Arsen Mkrtchyan over 4 yearsAre you using asp.net web api core?
-
haldo over 4 yearsAre you using Newtonsoft.Json or System.Text.Json?
-
Alessandro Albi over 4 yearsSorry, I didn't specify it... I use asp.net web api core and I use Newtonsoft.Json in the core API and System.Text.Json in Xamarin app
-
-
Faraz Babakhel almost 3 yearsWorked for me. Thanks