Append a new item to a list within a list
Solution 1
lst = [[] for _ in xrange(2)]
(or just [[], []]
). Don't use multiplication with mutable objects — you get the same one X times, not X different ones.
Solution 2
list_list = [[] for Null in range(2)]
dont call it list
, that will prevent you from calling the built-in function list()
.
The reason that your problem happens is that Python creates one list then repeats it twice. So, whether you append to it by accessing it either with list_list[0]
or with list_list[1]
, you're doing the same thing so your changes will appear at both indexes.
Solution 3
You can do in simplest way....
>>> list = [[]]*2
>>> list[1] = [2.5]
>>> list
[[], [2.5]]
Solution 4
list = [[]]
list.append([2.5])
or
list = [[],[]]
list[1].append(2.5)
Solution 5
As per @Cat Plus Plus dont use multiplication.I tried without it.with same your code.
>> list = [[],[]]
>> list[1].append(2.5)
>> list
>> [[],[2.5]]
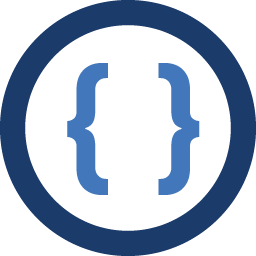
Admin
Updated on October 21, 2020Comments
-
Admin over 3 years
I'm trying to append a new float element to a list within another list, for example:
list = [[]]*2 list[1].append(2.5)
And I get the following:
print list [[2.5], [2.5]]
When I'd like to get:
[[], [2.5]]
How can I do this?
Thanks in advance.
-
agf almost 13 yearsI'd reccomend not using
_
as apparently it can mess up some interactive interpreters. Also, don't call itlist
, you're masking the builtin. Also, you'll get the same one X times for immutable objects too, it just doesn't often matter, unless you later want to check object identity. -
Cat Plus Plus almost 13 years@ag:
_
is standard "I don't care about this variable" variable. It is used by the interactive mode to store the last result, but it certainly doesn't mess anything up. -
agf almost 13 yearsI know what it's used for. I forget now, but I believe it messes up tracebacks in one of the IDEs.
-
orlp almost 13 years@agf: Then that is a bug of that IDE. Common codestyle is more important, to me, at least.
-
Niklas R almost 13 yearsBut allows you to create a list of any dimension.
-
Oben Sonne almost 13 yearsOf course! Over-engineered stuff usually lets you do "cool" things, but most of it is not needed with regard to the initial requirements ;-)
-
scraplesh over 12 years@CatPlusPlus underscore often used as translator in multilingual applications, so it's bad practice to use it unless you never work on such a projects
-
Dani over 7 yearsC'mon you can find so many more options. At the biggest case, use... I don't know.. max. (disclaimer: docs.python.org/2/library/functions.html#max)
-
Vinícius Figueiredo over 6 yearsA note since this question has many views: in Python3+, use
range(2)
instead ofxrange(2)
.