Argparse pass command line string to variable python3
Solution 1
There are several problems with this code, and with how you've used it.
The long option should be specified with two hyphens:
parser.add_argument('-u', '--username', help='The Username for authentication.')
Each option only populates one value in
args
, so you shouldn't try to unpack two. Try this instead:username = args.username
Values are separated on the command line by spaces, not commas, so use this:
--hostlist 192.168.1.1 192.168.1.2
Values on the command line that have spaces in them will be split on whitespace and interpreted as separate values ("words"). To force them to be interpreted as separate words, enclose them in quotes:
--commands 'uname -a' 'whoami'
Alternatively, you can escape the spaces with backslashes:
--commands uname\ -a whoami
Solution 2
it worked for me :
import optparse
parser = optparse.OptionParser('usage%prog'+'-username <username>'+'-password<pass> '+' -localhost<localhost>' +'-commands <commands> ')
parser.add_option('-u', dest='username', help='The Username for authentication.')
parser.add_option('-p', dest='password', help='The password for authentication.')
parser.add_option('-l', dest='hostlist', help='List of devices to interact with.')
parser.add_option('-c', dest='commands', help='An exact list of commands to run')
(options,args) = parser.parse_args()
username = options.username
password = options.password
hostlist = options.hostlist
commands = options.commands
print username,password,hostlist,commands
python ssh.py -u admin -p password -l '192.168.1.1, 192.168.1.2' -c 'uname -a',whoami
output:
admin password 192.168.1.1,192.168.1.2 uname -a,whoami
note 1: you can replace -username with -u in my code then you should type :
python ssh.py --username (and the other options)
note2: you used (192.168.1.1, 192.168.1.2) and (uname -a) because there is a space in ips and (-a) in (uname -a) your program has issue to fix it you have 2 way :
1) remove space use (uname-a) or (192.168.1.1,192.168.1.2)
2) use quotes ('192.168.1.1, 192.168.1.2') instead of (192.168.1.1, 192.168.1.2)
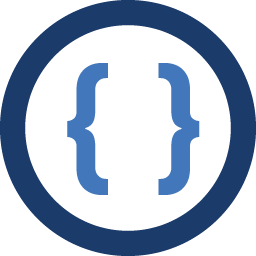
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
I've read the
argparse
docs and i am trying to make a program that takes command line arguments and passes those values to variables like so:ssh.py -username admin -password password -hostlist 192.168.1.1, 192.168.1.2 -commands uname -a, whoami
When I statically assign these values within my program it works, however I am unable to get argpase to pass strings into variables regardless of if the destination is a list or a single string.
This works:
hostlist = ['192.168.1.1','192.168.1.2'] username = 'admin' password = 'password' commands = ['uname -a','whoami']
This runs silently and does not work or generate an error or write a log file:
parser = argparse.ArgumentParser() parser.add_argument('-u', '-username', help='The Username for authentication.') parser.add_argument('-p', '-password', help='The password for authentication.') parser.add_argument('-l', '-hostlist', nargs='+', help='List of devices to interact with.') parser.add_argument('-c', '-commands', nargs='+', help='An exact list of commands to run') args = parser.parse_args() u,username = args.username p,password = args.password l,hostlist = args.hostlist c,commands = args.commands