Arguments of a constant creation must be constant expressions while implement a model using freezed
I ran into the same problem this morning. There's not a direct way that I could see to achieve this. However, a different approach to the problem may be in order.
Hopefully you are using a state management solution in your app. In my case it's RiverPod. So, rather than having the model know directly how to generate a default value for a property I elected to have my RiverPod state provider generate the value when creating the model.
So with a model like this...
@freezed class Pix with _$Pix {
const factory Pix({String? id, String? description, String? uri}) = _Pix;
}
...where I need the id to be generated (in my case it's a uuid), I have a StateNotifier handle that for me
class PixList extends StateNotifier<List<Pix>> {
PixList([List<Pix>? initialPixList]) : super(initialPixList ?? []);
void add(String description, String uri) {
state = [...state,
Pix(
id: _uuid.v4(),
description: description,
uri: uri
)
];
}
}
Since it's my state provider that should be handling creating objects, I'm effectively delegating responsibility for assigning that initial id value to the provider, not the model itself which remains nice and thin
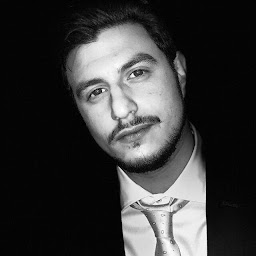
Comments
-
Stefano Saitta over 1 year
I'm having a small issue converting this class using
freezed
since is not possible to have a default value which is not constant, so the lineDateTime nocache= DateTime.now()
is not possible to be transformed into@Default(DateTime.now()) DateTime nocache
Here the full code
import 'package:equatable/equatable.dart'; abstract class DynamicLinkState extends Equatable { const DynamicLinkState(); @override List<Object> get props => []; } class DynamicLinkInitial extends DynamicLinkState { @override String toString() => 'DynamicLinkInitial'; } class DynamicLinkToNavigate extends DynamicLinkState { final String path; final DateTime nocache = DateTime.now(); DynamicLinkToNavigate({this.path}); @override List<Object> get props => [path, nocache]; @override String toString() => 'DynamicLinkToNavigate'; }
How can I eventually do that?
Additional context I'm using a
nocache
attribute here becausebloc
is optimize to not send the same event multiple times, but this is a valid use case in this situation since i might expect the user to receive more then one time the same dynamic link. So the solution we found is simply to invalidate this optimization by passing an always changingnocache
parameter.So a valid solution to this question might also be to simply remove this workaround in favor of a more solid solution.