Array Indexing in Python
Solution 1
The index
method does not do what you expect. To get an item at an index, you must use the []
syntax:
>>> my_list = ['foo', 'bar', 'baz']
>>> my_list[1] # indices are zero-based
'bar'
index
is used to get an index from an item:
>>> my_list.index('baz')
2
If you're asking whether there's any way to get index
to recurse into sub-lists, the answer is no, because it would have to return something that you could then pass into []
, and []
never goes into sub-lists.
Solution 2
list is an inbuilt function don't use it as variable name it is against the protocol instead use lst.
To access a element from a list use [ ] with index number of that element
lst = [1,2,3,4]
lst[0]
1
one more example of same
lst = [1,2,3,4]
lst[3]
4
Use (:) semicolon to access elements in series first index number before semicolon is Included & Excluded after semicolon
lst[0:3]
[1, 2, 3]
If index number before semicolon is not specified then all the numbers is included till the start of the list with respect to index number after semicolon
lst[:2]
[1, 2]
If index number after semicolon is not specified then all the numbers is included till the end of the list with respect to index number before semicolon
lst[1:]
[2, 3, 4]
If we give one more semicolon the specifield number will be treated as steps
lst[0:4:2]
[1, 3]
This is used to find the specific index number of a element
lst.index(3)
2
This is one of my favourite the pop function it pulls out the element on the bases of index provided more over it also remove that element from the main list
lst.pop(1)
2
Now see the main list the element is removed..:)
lst
[1, 3, 4]
For extracting even numbers from a given list use this, here i am taking new example for better understanding
lst = [1,1,2,3,4,44,45,56]
import numpy as np
lst = np.array(lst)
lst = lst[lst%2==0]
list(lst)
[2, 4, 44, 56]
For extracting odd numbers from a given list use this (Note where i have assingn 1 rather than 0)
lst = [1,1,2,3,4,44,45,56]
import numpy as np
lst = np.array(lst)
lst = lst[lst%2==1]
list(lst)
[1, 1, 3, 45]
Happy Learning...:)
Solution 3
In your second example, your list is going to look like this:
[0, 0, [1, 2, 3, 4], 0, 0]
There's therefore no element 4
in the list.
This is because when you set list[2]
, you are changing the third element, not updating further elements in the list.
If you want to replace a range of values in the list, use slicing notation, for example list[2:]
(for 'every element from the third to the last').
More generally, the .index
method operates on identities. So the following will work, because you're asking python where the particular list object you inserted goes in the list:
lst = [0]*5
lst2 = [1,2,3,4]
lst[2] = lst2
lst.index(lst2) # 2
Solution 4
The answer to your question is no, but you have some other issues with your code.
First, do not use list
as a variable name, because its also the name of the built-in function list
.
Secondly, list.index[4]
is different than list.index(4)
; both will give errors in your case, but they are two different operations.
Solution 5
If you want to pull the index of a particular element then index function will help. However, enumerate
will do similar to the dictionary example,
>>> l=['first','second','third']
>>> for index,element in enumerate(l):
... print index,element
...
output
0 first
1 second
2 third
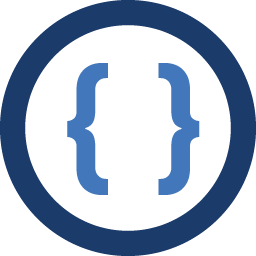
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Beginner here, learning python, was wondering something.
This gives me the second element:
list = [1,2,3,4] list.index(2) 2
But when i tried this:
list = [0] * 5 list[2] = [1,2,3,4] list.index[4]
I get an error. Is there some way to pull the index of an element from an array, no matter what list it's placed into? I know it's possible with dictionaries:
info = {first:1,second:2,third:3} for i in info.values: print i 1 2 3
Is there something like that for lists?