array of threads creation in c
Solution 1
pthread_create(&thread[i],NULL,student,(void*)&id1[i] );
if(i<6)
{
pthread_create(&thread[i],NULL,teacher,(void*)&id2[i]);
}
For i<6
, the value in thread[i]
for student
is overwritten by later pthread_create()
, which makes 6 threads leaked. 6 joinable threads remains running when main()
is about to return.
Solution 2
I don't think that your problem is in the end when joining threads, but in the beginning when threads are started.
First of all, id2[i]=i
might give a segfault unless that statement is moved to within the check that i<6
.
Next, overwriting the same &thread[i]
within the if-statement which was already created before the if-statement means that you have lost control of 6 student threads, those will not be joined at the end.
Solution 3
One of the approach to avoid the problem of undefined behavior due to id2[i]=i and also the issue of number of threads left without joining at end can be by having separate array for teacher/student coupled with appropriate changes in creation/joining procedure.
You can have separate array of pthread_t for student and teacher as below :
pthread_t thread_teacher[6];
pthread_t thread_student[25];
Accordingly, the update in creation and joining procedure for covering both student and teacher can be as below :
for(i=0;i<25;i++)
{
id1[i]=i;
pthread_create(&thread_student[i],NULL,student,(void*)&id1[i] );
if(i<6)
{
id2[i]=i;
pthread_create(&thread_teacher[i],NULL,teacher,(void*)&id2[i]);
}
}
for (i=0;i<25;i++)
{
pthread_join(thread_student[i],NULL);
if(i<6)
pthread_join(thread_teacher[i],NULL);
}
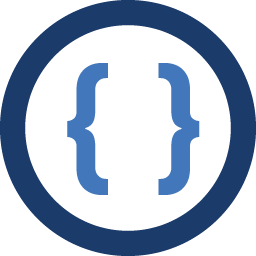
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I have created two array of threads using POSIX thread.There are two thread functions student and teacher. My sample program is given below. I have a small problem which I have commented out in the code . I have written a thread join function at the last of main function. Is it okey or is there anything wrong in writing it in this way?
Sample code fragment:
int main(void) { pthread_t thread[25]; int i; int id1[25]; //for students int id2[6]; //for teachers for(i=0;i<25;i++) { id1[i]=i; id2[i]=i; pthread_create(&thread[i],NULL,student,(void*)&id1[i] ); if(i<6) { pthread_create(&thread[i],NULL,teacher,(void*)&id2[i]); } } for (i=0;i<25;i++) { pthread_join(thread[i],NULL); //problem in this line } return 0;
}