Array.prototype.find() returns undefined in async function
16,876
Your are missing a return
. The predicate (the function passed to find) returns undefined in your case, so the find algorithm does not get a "match".
Just add the return
:
let found_user = response.data.find(acc=>{ //found_user is always undefined
return acc.username == user_to_find;
})
You can leave it out, if you are not using curly braces
let found_user = response.data.find(acc=> ( //found_user is always undefined
acc.username === user_to_find;
))
I'd recommend also to use ===
(strict comparison) instead of ==
for comparison.
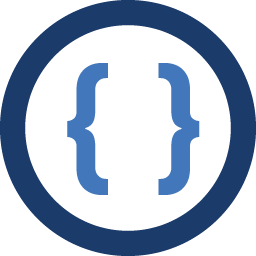
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have an issue with the
Array.prototype.find()
method. It doesn't seem to work properly when it is used in$http request..
I've tried everything that came to mind but it always returns undefined. I guess the bug is there because I loggedresponse.data
anduser_to_find
before definingfound_user
and their values are as expected.So I have this service:
app.service('UserService', ['$http','$q', function($http, $q) { return { findUser: function(user_to_find) { let defer = $q.defer(); $http({ method: "GET", url: 'http://localhost:3000/users' }).then(function(response){ let found_user = response.data.find(acc=>{ //found_user is always undefined acc.username == user_to_find; }) defer.resolve(found_user); }),function(response) { defer.reject(response); console.log("Error finding user"); } return defer.promise; } } }])
-
Admin almost 6 yearsOh my god. I feel so stupid, I thought that my mistake is in the promises.. I've been looking for it a whole day.. Thank you so much and sorry for the dumb question! I will follow your advice for the strict comparison, thank you!
-
lipp almost 6 yearsYou are welcome! Don't worry, everybody did have similar issues more than once :)