Array prototype is read only, properties should not be added no-extend-native
Solution 1
From EsLint Docs:
In JavaScript, you can extend any object, including builtin or “native” objects. Sometimes people change the behavior of these native objects in ways that break the assumptions made about them in other parts of the code.
For example here we are overriding a builtin method that will then affect all Objects, even other builtin.
// seems harmless
Object.prototype.extra = 55;
// loop through some userIds
var users = {
"123": "Stan",
"456": "David"
};
// not what you'd expect
for (var id in users) {
console.log(id); // "123", "456", "extra"
}
In-short, Array.prototype.inState
will extend the array.prototype
and hence whenever you want to use an array, the instate function will be added to that array too.
So, in your case this example will be applied to array.
Array.prototype.inState = function (needle, haystack) {
let index = this.findIndex(value => value[needle] === haystack);
return index === -1;
};
// loop through some userIds
var users = [{"123": "Stan"},{"456": "David"}];
// not what you'd expect
for (var id in users) {
console.log(users[id]); // "123", "456", "extra"
}
WorkAround
You can add this line to ignore warning.
/*eslint no-extend-native: ["error", { "exceptions": ["Object"] }]*/ to ignore that warning.
Ref: https://eslint.org/docs/rules/no-extend-native
Solution 2
Its because esLint gets it mutation to the native protoTypes chain.
You can add // eslint-disable-next-line no-extend-native
in above the line it should be fine.
Related videos on Youtube
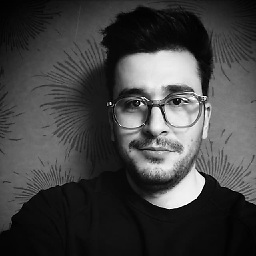
AH.Pooladvand
Updated on October 09, 2022Comments
-
AH.Pooladvand about 1 year
So basically I have this code:
Array.prototype.inState = function (needle, haystack) { let index = this.findIndex(value => value[needle] === haystack); return index === -1; };
and it works fairly enough to check if the given needle is exists in the react state or not. but ESlint keep saying that:
Array prototype is read only, properties should not be added no-extend-native
so my question is: What is wrong with my code ?
-
Reinier Garcia about 2 yearsWith: /*eslint no-extend-native: ["error", { "exceptions": ["Object"] }]*/ ... It shows an error: "Failed to compile ./src/components/List/List.js Line 8: Array prototype is read only, properties should not be added no-extend-native Search for the keywords to learn more about each error." The proper solution/workaround is adding just: // eslint-disable-next-line no-extend-native ...as Ashif Zafar said.