array_map - "Argument passed must be of the type array, string given" error
12,904
Solution 1
Your function del_space
takes an array as the argument. array_map
takes every element of the array (second argument) itself, and sends it to the callback (first argument). So unless if you have an array of arrays, this would not work. Your example should look like this:
$array = ['lblab; la'];
function del_space($a){
return preg_replace("/; +/", "", $a);
}
$no_space = array_map("del_space", $array);
print_r($no_space);
Gives output:
Array ( [0] => lblab;la)
If you want to pass an array of arrays, than the input should look like this:
$array = [
['blabla; bla'],
['blabla2; bla2'],
];
function del_space(array $a){
foreach($a as $key => $value){
$a[$key] = preg_replace("/; +/", "", $value);
}
return $a;
}
$no_space = array_map("del_space", $array);
print_r($no_space);
With the output:
Array ( [0] => Array ( [0] => blablabla ) [1] => Array ( [0] => blabla2bla2 ) )
Solution 2
array_map()
loops $array
for you and since so I am assuming that each item inside of $array
is not an array object but del_space()
requires an array to be passed in to it.
It sounds like you have:
$array = array( 'some item' ); // Fails
but you need something like:
$array = array( array( 'some item' ) ); // Success
if you wish to use array_map()
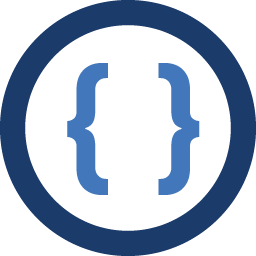
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am not sure why the following does not work. I get the above error (topic):
$array = array (); //something goes in here function del_space(array $a){ foreach($a as $key => $value){ preg_replace("/; +/", "", $value); } } $no_space = array_map("del_space", $array);
-
Script47 almost 6 yearsWhat line? The error seems self-explanatory.
-
Admin almost 6 yearsI pass it an array and it gives me the message that this is a string. I check that with is_array() one line above the code. This is a shortened version. This is taken from what I am writing in an IDE.
-
Liam G almost 6 yearsthe array is empty?
-
Admin almost 6 yearseval.in/1020730 - Check it out.
-
Mawia HL almost 6 yearsIf you are looking nospace in the array, just use the built function trim();
-
Sammitch almost 6 yearsPost your actual code in your actual question. The code you linked to on eval.in has an additional statement that is the source of your error.
-
-
Admin almost 6 yearsCheck out the eval.in url. It is an array and it does not work.
-
MonkeyZeus almost 6 years@gs3 I have a meeting, I'll check later.
-
Admin almost 6 yearsGot it, thanks! This is the very first day when I write code, but after like 11 months of the theoretical edu. Thanks! How long to get better / feel the progress, if I can ask?
-
spielerds almost 6 yearsYou're welcome, I'm glad you got it working. It's hard to tell, I think with PHP it is relatively easy to learn the basics, but there is something to learn every day :) php.net is a great starting point, there is detailed description for every function with many examples.