ask user for permission to show alert when firing local notification
Solution 1
add this code, it will show a alert view to ask user for permission.
if ([UIApplication instancesRespondToSelector:@selector(registerUserNotificationSettings:)]) {
[[UIApplication sharedApplication] registerUserNotificationSettings:[UIUserNotificationSettings settingsForTypes:UIUserNotificationTypeAlert|UIUserNotificationTypeSound|UIUserNotificationTypeBadge
categories:nil]];
}
you can add this code in application:didFinishLaunchingWithOptions; method, so that the app will ask your user when they launch the app, or you can add this code when you set Local Notification, it's up to you.
Solution 2
蘇健豪's answer is good.
In Swift it looks like this:
let registerUserNotificationSettings = UIApplication.instancesRespondToSelector("registerUserNotificationSettings:")
if registerUserNotificationSettings {
var types: UIUserNotificationType = UIUserNotificationType.Alert | UIUserNotificationType.Sound
UIApplication.sharedApplication().registerUserNotificationSettings(UIUserNotificationSettings(forTypes: types, categories: nil))
}
Also see here: Ask for User Permission to Receive UILocalNotifications in iOS 8
Solution 3
In the swift language....
var type = UIUserNotificationType.Badge | UIUserNotificationType.Alert | UIUserNotificationType.Sound;
var setting = UIUserNotificationSettings(forTypes: type, categories: nil);
UIApplication.sharedApplication().registerUserNotificationSettings(setting);
UIApplication.sharedApplication().registerForRemoteNotifications();
Solution 4
//register notifications
if([application respondsToSelector:@selector(registerUserNotificationSettings:)]) //ios 8+
{
[application registerUserNotificationSettings:[UIUserNotificationSettings settingsForTypes:UIUserNotificationTypeAlert|UIUserNotificationTypeBadge|UIUserNotificationTypeSound categories:nil]];
[application registerForRemoteNotifications];
}
else // ios 7 or less
{
[application registerForRemoteNotificationTypes:UIRemoteNotificationTypeSound | UIRemoteNotificationTypeAlert | UIRemoteNotificationTypeBadge];
}
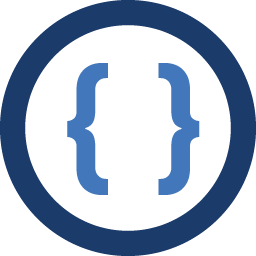
Admin
Updated on June 07, 2022Comments
-
Admin about 2 years
I want to show an alert when the local notification is fired, but for that I have to ask for permission, as it says to me when I run the app on my iPhone:
Attempting to schedule a local notification {fire date = Friday 13 June 2014 12 h 10 min 27 s Central European Summer Time, time zone = (null), repeat interval = 0, repeat count = UILocalNotificationInfiniteRepeatCount, next fire date = Friday 13 June 2014 12 h 10 min 27 s Central European Summer Time, user info = (null)} with an alert but haven't received permission from the user to display alerts
How can I do that? Here´s the code as it is now:
UILocalNotification *localNotif = [[UILocalNotification alloc] init]; localNotif.fireDate = [[NSDate date] dateByAddingTimeInterval:timeUntilNotification]; localNotif.soundName = UILocalNotificationDefaultSoundName; localNotif.alertBody = @"ZEIT!"; localNotif.alertAction = @"Show me the Timer!"; localNotif.applicationIconBadgeNumber = [[UIApplication sharedApplication] applicationIconBadgeNumber] +1; [[UIApplication sharedApplication] scheduleLocalNotification:localNotif];
-
LargeGlasses over 9 yearsThis snippet is great, thank you! Note to everyone: put good thought into when specifically in your app you want to ask the user permission to send notifications, because you don't want to scare them off right when they open your app. Build some trust first, then ask their permission. UX food for thought :)
-
Morkrom over 9 yearsIn swift it looks like this: let registerUserNotificationSettings = UIApplication.instancesRespondToSelector("registerUserNotificationSettings:") if registerUserNotificationSettings { var types: UIUserNotificationType = UIUserNotificationType.Alert | UIUserNotificationType.Sound UIApplication.sharedApplication().registerUserNotificationSettings(UIUserNotificationSettings(forTypes: types, categories: nil))}
-
samthui7 almost 9 yearsThis snippet is recommended for iOS 8 and above.
-
TomSawyer about 8 yearsCan i do a re-ask? If user accidentally don't allow the permission, can i make a button and if user tap on it the app will re-ask for the notification permission?