ASP.NET C# How to fill table from database
10,661
You should use a DataControl like a asp:GridView
instead of a asp:Table
. In case of a asp:Table
you have to add the whole data maually to your Rows / Columns. In case of a asp:GridView
it's just a databinding.
cs:
string query = "SELECT name, startdate FROM table WHERE startdate > @end_date AND name = ...";
using (SqlConnection myConnection = new SqlConnection(ConnectionString))
{
using (SqlCommand cmd = new SqlCommand(query, myConnection))
{
myConnection.Open();
SqlDataReader dr = cmd.ExecuteReader(CommandBehavior.CloseConnection);
DataTable dt = new DataTable();
dt.Load(dr);
Tournament.DataSource = dt;
Tournament.DataBind();
}
}
aspx:
<asp:GridView ID="Tournament" runat="server"></asp:GridView>
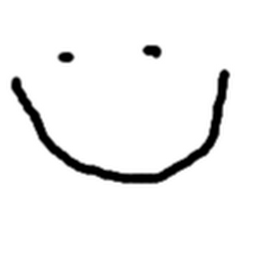
Author by
Craiten
Updated on June 04, 2022Comments
-
Craiten almost 2 years
This is my table in my .aspx file:
<asp:Table ID="Tournament" runat="server"> <asp:TableHeaderRow> <asp:TableHeaderCell>Name</asp:TableHeaderCell> <asp:TableHeaderCell>Start date</asp:TableHeaderCell> </asp:TableHeaderRow> </asp:Table>
This is how I get the data from my database:
string conStr = "..."; SqlConnection sqlConnection = new SqlConnection(conStr); string sqlString = "SELECT name, startdate FROM table WHERE startdate > @end_date AND name = ..."; IDbCommand idbCommand = new SqlCommand(sqlString, sqlConnection); IDbDataParameter parameter = idbCommand.CreateParameter(); parameter.ParameterName = "@end_date"; parameter.Value = DateTime.Now; idbCommand.Parameters.Add(parameter); sqlConnection.Open();
How do I fill my asp:Table with the data from my sql databae?
-
Dexter Whelan almost 4 yearswhat is gridview in this context? why use that over just having table as <asp:Table ID="LinkResults" runat="server"></asp:Table> ?
-
fubo almost 4 years@DexterWhelan
Tournament.DataSource = dt;
here I assign theDataTable
to the GridView