Asp.net core Identity successful login redirecting back to login page
Solution 1
In order to get the ASP.NET Core pipeline to recognise that a user is signed in, a call to UseAuthentication
is required in the Configure
method of your Startup
class, like so:
app.UseAuthentication();
app.UseMvc(); // Order here is important (explained below).
Using the Cookies authentication scheme, the use of UseAuthentication
loosely performs the following:
- Reads the content of the
.AspNetCore.Identity.Application
cookie from the request, which represents the identity of the user making the request. - Populates the
User
property ofHttpContext
with aClaimsPrincipal
that represents said user.
This is a simplified explanation of what happens, but it highlights the important job that the authentication middleware performs. Without the authentication middleware, the .AspNetCore.Identity.Application
will not be used for authenticating the user and therefore the user will not be authenticated. In your case, although the user has signed in (i.e. the cookie is being set), the pipeline middleware (e.g. MVC) does not see this user (i.e. the cookie is not being read) and so sees an unauthenticated request and redirects again for login.
Given that the authentication middleware reads the cookie and subsequently populates the ClaimsPrincipal
, it should be clear that the UseAuthentication
call must also be before the UseMvc
call in order for this to occur in the correct order. Otherwise, the MVC middleware runs before the Authentication middleware and will not be working with a populated ClaimsPrincipal
.
Why is it failing to login if you don't add the middleware that handles the login?!?
The middleware doesn't handle the login - it handles the authentication process. The user has logged in, which is confirmed by the presence of the .AspNetCore.Identity.Application
cookie. What is failing here is the reading of said cookie.
Solution 2
Note that the order is IMPORTANT. I had these reversed and experienced the exact same problem the original poster experienced. Hours wasted before I figured this out...
app.UseAuthentication();
app.UseAuthorization();
Solution 3
In addition to @Kirk Larkin answer if you use .net core 3 (in this time is preview version)
put your "app.UseEndpoints" in startup.cs to end of the code block.
for example it should be in this order
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
endpoints.MapRazorPages();
});
Solution 4
app.UseAuthentication(); app.UseMvc();
This was already in my code.However I was facing same issue. But the problem was with the Chrome browser on my case.It was working with other browser like mozilla. It starts to work on Chrome too, after i cleared all the cookies and cashes.
Solution 5
I had to do a couple of these solutions.
First, I had app.UseAuthentication(); and app.UseAuthorization(); in the incorrect order.
Second, After making the adjustment to startup.cs I was still experiencing the same problem. Doing a hard refresh a few times fixed it (can also just clear the browser cache).
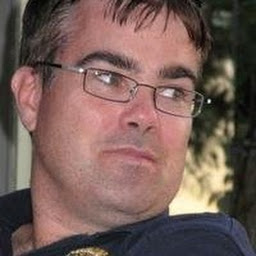
Jero
Updated on July 15, 2022Comments
-
Jero almost 2 years
I have a problem where the asp.net identity framework is redirecting the user back to the login page after they have logged in successfully.
This is using the standard Asp.net Core Identity. It is the version 2.1.1. The scaffolding that generates the razor pages. Not sure if that is significant.
I know the user is successfully logging in because I get the log message
...Areas.Identity.Pages.Account.LoginModel: Information: User logged in.
But then it redirects straight back to the login page.
If I use fiddler I can see that there is a cookie on the request so it all looks good from that perspective.
.AspNetCore.Identity.Application=CfDJ8KJxkuir9ZJIjFLCU2bzm9n6X...
So I guess the middleware that is handling the authentication but not accepting the cookie?
If I could see what the actual middleware for the auth was doing I might have an idea but I can't find it.
Any help appreciated