ASP.Net MVC 4 Set 'onsubmit' on form submission
30,257
You need this instead:
@using (Html.BeginForm("ActionMethod","Controller",FormMethod.Post, new { onsubmit = "return myJsFunction()" }))
{
//form
}
Notice the using
this makes the form self closing, without the using you need to close it as detailed in this MSDN article.
You can confirm javascript is called with this to isolate the problem:
@using (Html.BeginForm("ActionMethod","Controller",FormMethod.Post, new { onsubmit = "alert('test')" }))
{
<input type="submit" value="test" />
}
This should pop up an alert.
If the first one fails and the second one works, there is a problem with your js script references. This would raise an error in your browser console.
Update
Instead of binding your form obtrusively, if you give your form an Id you could use the following jquery instead (jQuery reference):
@using (Html.BeginForm("ActionMethod","Controller",FormMethod.Post, new { id = "target"}))
{
//form
}
<script>
$(function () {
$("#target").submit(function (event) {
event.preventDefault();
myJsFunction();
});
});
</script>
This would bind when the form when the document is ready.
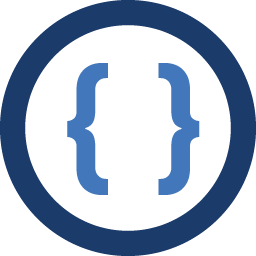
Author by
Admin
Updated on July 12, 2022Comments
-
Admin almost 2 years
I have the following form:
@Html.BeginForm("ActionMethod","Controller",FormMethod.Post)
On submission I want to run a Javascript function, so I added the following:
@Html.BeginForm("ActionMethod","Controller",FormMethod.Post, new { onsubmit = "myJsFunction()" })
But, it doesn't work... What am I doing wrong? Thanks!