ASP.Net MVC How to display an image property of a model in a view?
Solution 1
You need to write a controller action that will write the image into the response stream and set the proper content type to say "image/png". Then you can use the img
tag to reference this action in your view:
public ActionResult Image()
{
byte[] image = GenerateImage();
return File(image, "image/png");
}
And inside your view:
<img src="<%= Url.Action("Image") %>" alt="" />
Solution 2
You will need a HttpHandler if you want to do things like this.
http://dotnetperls.com/ashx-handler
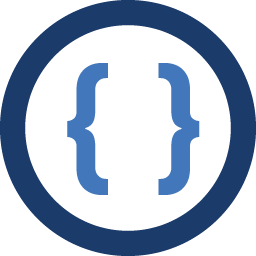
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
I'm new to asp.net mvc, but I'm trying to do an funny app with GDI+ and I need to do some image view with asp.net mvc.
I have a Model which has an image property:
namespace DomainModel.Entities { public class BackgroundImage { // Properties public Image Image {get; private set; } public BackgroundImage() { Image = Image.FromFile(@"D:\ProjectZero\DomainModel\image\bkg.PNG"); } } }
The controller sends to the view the following:
public ActionResult Index() { BackgroundImage bkImg = new BackgroundImage(); return View(bkImg); }
The view looks like this:
<p> Height: </p><%= Model.Image.Height //it prints the height of the image %> </br> <p> Width: </p><%= Model.Image.Width //it prints the width %> </br> <p> Image: </p><%= Model.Image //does not work to display the image %>
How do I display that image property?
I need that background image for a div. I do not need to resize the image, I only need to display it in it's normal size.
Is there a Html Helper that I miss?
Thank you, for your time!
-
Admin over 14 yearsThis solved my problem, but I needed to use this: public byte[] imageToByteArray(System.Drawing.Image imageIn) { MemoryStream ms = new MemoryStream(); imageIn.Save(ms,System.Drawing.Imaging.ImageFormat.Gif); return ms.ToArray(); } for converting my image into a byte array.
-
Andy Clark about 12 yearsI have been trying to do this for 2 days and have been led down the path of HttpHelpers, derived ActionResults called ImageResults and has got me no where, this solution worked first time, Excellent!!
-
kroiz over 10 yearsThe At sign is missing in the view code example above. <img src="@Url.Action("Image") " />
-
Darin Dimitrov over 10 years@kroiz, that's because the example given here is using the WebForms view engine which doesn't have such notion as the
@
sign in Razor. In the WebForms view engine you are using<%
and%>
to render server side code.