Assign values to array during loop - Python
Appending elements while looping using append()
is correct and it's a built-in method within Python lists
.
However you can have the same result:
Using list comprehension:
result_t = [k for k in range(1,6)]
print(result_t)
>>> [1, 2, 3, 4, 5]
Using +
operator:
result_t = []
for k in range(1,6):
result_t += [k]
print(result_t)
>>> [1, 2, 3, 4, 5]
Using special method __iadd__
:
result_t = []
for k in range(1,6):
result_t.__iadd__([k])
print(result_t)
>>> [1, 2, 3, 4, 5]
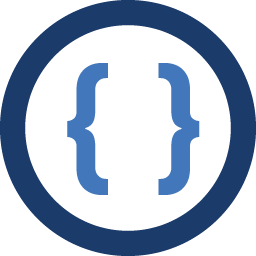
Admin
Updated on December 11, 2021Comments
-
Admin over 2 years
I am currently learning Python (I have a strong background in Matlab). I would like to write a loop in Python, where the size of the array increases with every iteration (i.e., I can assign a newly calculated value to a different index of a variable). For the sake of this question, I am using a very simple loop to generate the vector
t = [1 2 3 4 5]
. In Matlab, programming my desired loop would look something like this:t = []; for i = 1:5 t(i,1) = i; end
I have managed to achieve the same thing in Python with the following code:
result_t = [] for i in range(1,5): t = i result_t.append(t)
Is there a more efficient way to assign values to an array as we iterate in Python? Why is it not possible to do
t[i,1] = i
(error: list indices must be integers or slices, not tuple) ort.append(t) = t
(error: 'int
' object has no attribute 'append')?Finally, I have used the example above for simplicity. I am aware that if I wanted to generate the
vector [1 2 3 4 5]
in Python, that I could use the function "np.arange(1,5,1)
"Thanks in advance for your assistance!
-> My real intention isn't to produce the vector [1 2 3 4 5], but rather to assign calculated values to the index of the vector variable. For example:
result_b = [] b = 2 for i in range(1,5): t = i + b*t result_b.append(t)
Why can I not directly write
t.append(t)
or use indexing (i.e.,t[i] = i + b*t
)?