Assigning values to a public byte array in one line C#
Solution 1
Your first example is fine but it may only be used in a declaration. The elements must be implicitly convertible to the element type. The size is determined from the number of elements given.
byte[] foo = { 0x32, 0x00, 0x1E, 0x00 };
Alternatively you can also d this
byte[] foo = new byte[4];
foo[0] = 0x32;
foo[1] = 0x00;
foo[2] = 0x1E;
foo[3] = 0x00;
as stated above that the syntax that you are trying to use can only be used in a declaration. so try like this.
public byte[] SetSpeed;
private void trackBar1_Scroll(object sender, EventArgs e)
{
if (trackBar1.Value == 0)
{
try
{
stop = true;
UpdateSThread.Abort();
Thread.Sleep(350);
}
catch { }
//note it will always create a new array
SetSpeed = new byte[]{0x00,0x00,0x00,0x00};
WriteMem(GetPlayer() + STATUS_OFFSET, SetSpeed);
label1.Text = "Normal";
}
}
Solution 2
You can only use the form where you don't specify new
when you're declaring a variable. So this is fine:
byte[] x = { 1, 2, 3 };
But this isn't:
byte[] x;
x = { 1, 2, 3 };
Instead you need something like:
byte[] x;
x = new byte[] { 1, 2, 3 };
Or for cases where type inference works in your favour and you're using C# 3 or higher, you can use an implicitly typed array:
string[] x;
x = new[] { "a", "b", "c" };
However, you really need to decide whether you want to create a new array, or whether you want to mutate the existing array. Given that you're just setting all the values to 0, you could instead use:
Array.Clear(SetSpeed, 0, 4);
EDIT: Now that the question contains this:
I would like to change values in an existing array,
then using
SetSpeed = new byte[] { ... }
is not appropriate, as that will change the value of SetSpeed
to refer to a different array.
To update an existing array, you could either just stick with the 4 separate statements, or perhaps extract it to a separate method with 4 parameters. It's not really clear what the values are going to be, which makes it hard to give you the best possible code.
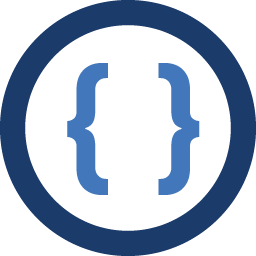
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am working with a public byte array and would like to assign values, like I assign arrays inside a method, i.e
byte[] foo = {0x32, 0x00, 0x1E, 0x00};
but when I define the values I am forced to do
foo[0] = 0x32; foo[1] = 0x00; foo[2] = 0x1E; foo[3] = 0x00;
If I use the first example VS gives an error "Only assignment, call, increment, decrement, await, and new object expressions can be used as a statement"
If it helps any, the array is always 4 bytes.
my code
public byte[] SetSpeed = new byte[4]; private void trackBar1_Scroll(object sender, EventArgs e) { if (trackBar1.Value == 0) { try { stop = true; UpdateSThread.Abort(); Thread.Sleep(350); } catch { } SetSpeed = {0x00,0x00,0x00,0x00}; WriteMem(GetPlayer() + STATUS_OFFSET, SetSpeed); label1.Text = "Normal"; } }
-
Jon Skeet almost 11 yearsThere's no need to do this though - the original code compiles anyway.
-
Admin almost 11 yearsThank you, this works, I guess I came across to vague, the public tag was relevant, but removed. no worries, I got my answer
-
Admin almost 11 yearsThank you for the advice on the Array.Clear, the next tick on the track bar, does start adding values > 0.
-
Jon Skeet almost 11 years@user2340475: But you still need to decide on whether you want a new array or not. Does anything else have a reference to the array already?
-
Jeppe Stig Nielsen almost 11 yearsIt is either
Array.Clear(SetSpeed, 0, 4);
or((IList<byte>)SetSpeed).Clear();
. -
Jon Skeet almost 11 years@JeppeStigNielsen: Thanks, fixed.
-
Admin almost 11 yearsNo it does not, when the track bar moves off 0, A thread is started to loop update the value in memory, the value being SetSpeed, when brought back to 0 the thread is exited and the value in memory is set back to 4 bytes of 0
-
Jon Skeet almost 11 years@user2340475: So what actually needs it as an array other than the method itself? It looks like you could just create it before calling WriteMem.
-
Admin almost 11 years'void UpdateSpeed() { stop = false; while (stop == false) { if (ReadMem(GetPlayer() + STATUS_OFFSET, 4) != SetSpeed) { WriteMem(GetPlayer() + STATUS_OFFSET, SetSpeed); } Thread.Sleep(300); } } not sure how to display this as code. but that is the thread that is started.
-
Jon Skeet almost 11 years@user2340475: Well you're comparing a byte array with a reference comparison there, so that's unlikely to work either... and unless
stop
is volatile, you may well not see changes from another thread...