Async arrow function expected no return value
Solution 1
Change the first line from if (!userAuth) return
to if (!userAuth) return {}
, this should do the trick
Explanation
The error you're having says consistent-return
at the end, which basically means your function should consistently return the same type.
With that in mind, if we take a look at the first line in your function
if (!userAuth) return;
this line returns a void
, but the last line in the function is returning some other type
return userRef;
userRef
is definitely not of type void
and this is why you are having this error.
Solution 2
The error message is telling you that an arrow function should always or never return a value. Look here
You have if (!userAuth) return;
and return userRef;
Change if (!userAuth) return {};
should fix the problem.
Solution 3
There is a violation of the eslint 'consistent-return' rule in the code.
This code:
if (!userAuth) return;
returns undefined, while the last line of the arrow function:
return userRef;
};
returns userRef
.
The fix is to return something that isn't undefined in the first case.
Solution 4
try to replace return to return undefined
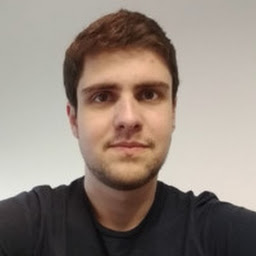
Augusto Mallmann
Updated on June 17, 2022Comments
-
Augusto Mallmann about 2 years
I'm building an application with Firebase oAuth. I followed all the instructions, but my code is returning an error saying that 'asyn arrow function expected no return value'.
I saw that there are multiple posts with the same title, but have not found an answer.
import firebase from 'firebase/app'; import 'firebase/firestore'; import 'firebase/auth'; export const auth = firebase.auth(); export const firestore = firebase.firestore(); const config = { apiKey: 'AIzaSyDptaU9-hQIIpAW60S1-aGUSdcQdijbNAQ', authDomain: 'ecommerce-50be3.firebaseapp.com', projectId: 'ecommerce-50be3', storageBucket: 'ecommerce-50be3.appspot.com', messagingSenderId: '948534790840', appId: '1:948534790840:web:6329c3edcc717138a5424d', }; firebase.initializeApp(config); export const createUserProfileDocument = async (userAuth, additionalData) => { if (!userAuth) return; const userRef = firestore.doc(`users/${userAuth.uid}`); const snapShot = await userRef.get(); if (!snapShot.exists) { const { displayName, email } = userAuth; const createdAt = new Date(); try { await userRef.set({ displayName, email, createdAt, ...additionalData, }); } catch (error) { console.log('error creating user', error.message); } } return userRef; }; const provider = new firebase.auth.GoogleAuthProvider(); provider.setCustomParameters({ prompt: 'select_account' }); export const signInWithGoogle = () => auth.signInWithPopup(provider); export default firebase;