AsyncTask in a Loop In Android
17,692
Instead of Keeping AsynChronous Task into Loop, Keep Loop inside AsynChronous. and use onProgressUpdate
to update UI.
Updated for Example Code
public class Asyn extends AsyncTask<String, String, String> {
@Override
protected String doInBackground(String... params) {
for (String location : params) {
String tmp = getTemp(location);
publishProgress(tmp); /** Use Result **/
}
return null;
}
/**
* Calculate Weather
*
* @param location
* @return
*/
private String getTemp(String location) {
return location;
}
@Override
protected void onProgressUpdate(String... values) {
super.onProgressUpdate(values);
Log.e("Temo", values[0]);
}
}
And Pass the array of Location where you start process
Asyn m = new Asyn();
String arrryaLocation = null; // your vales
m.execute(arrryaLocation);
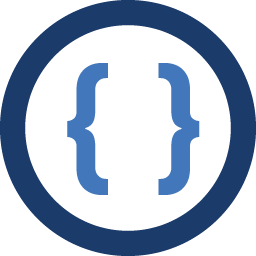
Author by
Admin
Updated on June 05, 2022Comments
-
Admin about 2 years
I am creating a app that will update the weather details of the city.The loop will be a List of City .So i want as much the cities are in there in the List.I will use the AsyncTask method to send the request and parse it.And at the same time i am inflating a layout and i have to put all the weather details corresponding to the city in the Layout .How could i achieve this please help me in this.
XML file that i am inflating
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/depart_details" android:layout_width="wrap_content" android:layout_height="wrap_content" > <ImageView android:id="@+id/flight_depart_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:padding="3dip" android:layout_marginTop="10dp" android:src="@drawable/f1" /> <TextView android:id="@+id/depart_time" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dp" android:layout_marginTop="5dp" android:layout_toRightOf="@+id/flight_depart_image" android:text="" android:textColor="#666666" android:textSize="25sp" android:textStyle="bold" /> <TextView android:id="@+id/depart_airport_city" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dp" android:layout_marginTop="5dp" android:layout_toRightOf="@+id/depart_time" android:text="" android:textColor="#666666" android:textSize="15sp" android:textStyle="bold" /> <TextView android:id="@+id/depart_airport" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/depart_airport_city" android:layout_marginLeft="125dp" android:text="N/A" android:textColor="#666666" android:textSize="12sp" android:textStyle="bold" /> <ImageView android:id="@+id/weather_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft ="40dp" android:layout_toRightOf="@+id/depart_airport" android:src="@drawable/image" /> <TextView android:id="@+id/tempraturetext" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="3dp" android:layout_toRightOf="@+id/weather_image" android:text="Temp:" /> <TextView android:id="@+id/temprature" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="3dp" android:layout_toRightOf="@+id/tempraturetext" android:text="20℃" /> <TextView android:id="@+id/humidity_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/temprature" android:layout_marginLeft="3dp" android:layout_toRightOf="@+id/weather_image" android:text="Humidity:" /> <TextView android:id="@+id/humidity" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/temprature" android:layout_marginLeft="3dp" android:layout_toRightOf="@+id/humidity_text" android:text="32" /> <LinearLayout android:layout_width="match_parent" android:layout_height="1dp" android:layout_below="@+id/depart_airport" android:layout_marginTop="5dp" android:background="#d3d3d3" > </LinearLayout>
this function i have created to send the request
private WeatherResponse requestWeatherUpdate(String location) { url = "" + location; //This location will be dyanamic and multiple Log.d("URL for Weather Upadate", url); WeatherUpdate weatherReq = new WeatherUpdate(new CallBack() { @Override public void run(Object result) { try { AppResponse = (String) result; response = ParseWeatherResponseXML .parseMyTripXML(AppResponse); } catch (Exception e) { Log.e("TAG Exception Occured", "Exception is " + e.getMessage()); } } }); weatherReq.execute(url); return response; }
AsynkTask
public class WeatherUpdate extends AsyncTask<String, Void, String> { Context context; CallBack callBack; public WeatherUpdate(CallBack callBack) { this.callBack = callBack; } @Override protected String doInBackground(String... arg0) { String responseString = ""; HttpClient client = null; try { client = new DefaultHttpClient(); HttpGet get = new HttpGet(IweenTripDetails.url); client.getParams().setParameter("http.socket.timeout", 6000); client.getParams().setParameter("http.connection.timeout", 6000); HttpResponse responseGet = client.execute(get); HttpEntity resEntityGet = responseGet.getEntity(); if (resEntityGet != null) { responseString = EntityUtils.toString(resEntityGet); Log.i("GET RESPONSE", responseString.trim()); } } catch (Exception e) { Log.d("ANDRO_ASYNC_ERROR", "Error is " + e.toString()); } Log.d("ANDRO_ASYNC_RESPONSE", responseString.trim()); client.getConnectionManager().shutdown(); return responseString.trim(); } @Override protected void onPostExecute(String result) { // TODO Auto-generated method stub super.onPostExecute(result); callBack.run(result); } }
-
Biraj Zalavadia over 10 yearsthis should be comment.
-
Biraj Zalavadia over 10 yearsthis should be comment
-
DropAndTrap over 10 yearsrun a loop inside the doInBackground
-
Admin over 10 years@sameer please elaborate more how could i do this by your way
-
Admin over 10 yearsSee i have to infalate the XML as well as with in the loop and have to put the values that i will recive from the response .How i have to do that i am not getting that
-
Tofeeq Ahmad over 10 yearsuse every line of code inside private String getTemp(String location) { return location; }
-
Tofeeq Ahmad over 10 yearsand you can change you method name, return type, paramter according to your requiremen
-
Admin over 10 yearsPlease comment on my code where i have to make the chages i am not geeting you
-
Admin over 10 years@Sameer please help me in this ...I need help
-
Lucia Belardinelli about 9 yearsI think this comment should be added in the answer :)