Attempt to invoke virtual method 'java.lang.Object android.content.Context.getSystemService(java.lang.String)' on a null object reference
Solution 1
LayoutInflater li = LayoutInflater.from(getBaseContext());
First, you do not need getBaseContext()
, nor do you need LayoutInflater.from()
. Use getLayoutInflater()
.
Second, you cannot call methods on the Activity
superclass until after super.onCreate()
, except in certain situations. Please postpone your initialization of promptsView
until after super.onCreate()
has been called.
Solution 2
View view = inflater.inflate(R.layout.fragment_name, container, false);
view.getContext();
Use view.getContext()
wherever you need Application Context in fragments.
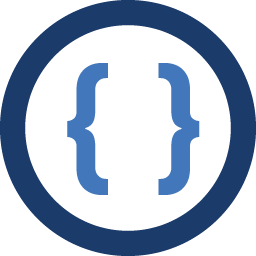
Admin
Updated on January 18, 2020Comments
-
Admin over 4 years
I am trying to check if my application is launched for the first time. If yes, the user is asked for an input. Then, check if Wi-Fi is connected. If Wi-Fi is connected, I use the input provides by the user to load a WebView.
But, the app crashes on launch with an error
Attempt to invoke virtual method 'java.lang.Object android.content.Context.getSystemService(java.lang.String)' on a null object reference
Code
public class MainActivity extends Activity { ProgressBar progressbar; WebView webView; LayoutInflater li = LayoutInflater.from(getBaseContext()); View promptsView = li.inflate(R.layout.propmt, null); String URL; protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); this.getWindow().requestFeature(Window.FEATURE_PROGRESS); setContentView(R.layout.activity_main); Boolean isFirstRun = getSharedPreferences("PREFERENCE", MODE_PRIVATE) .getBoolean("isFirstRun", true); if (isFirstRun) { otherWork(promptsView); } getSharedPreferences("PREFERENCE", MODE_PRIVATE).edit() .putBoolean("isFirstRun", false).commit(); ConnectivityManager connManager = (ConnectivityManager) getSystemService(CONNECTIVITY_SERVICE); NetworkInfo mWifi = connManager.getNetworkInfo(ConnectivityManager.TYPE_WIFI); if (mWifi.isConnected()) { getWindow().setFeatureInt( Window.FEATURE_PROGRESS, Window.PROGRESS_VISIBILITY_ON); webView = (WebView) findViewById(R.id.webView); webView.getSettings().setBuiltInZoomControls(true); webView.getSettings().setDisplayZoomControls(false); webView.getSettings().setRenderPriority(RenderPriority.HIGH); webView.getSettings().setCacheMode(WebSettings.LOAD_NO_CACHE); if (Build.VERSION.SDK_INT >= 19) { webView.setLayerType(View.LAYER_TYPE_HARDWARE, null); } else { webView.setLayerType(View.LAYER_TYPE_SOFTWARE, null); } webView.loadUrl(URL); webView.setWebViewClient(new WebViewClient()); WebSettings webSettings = webView.getSettings(); webSettings.setJavaScriptEnabled(true); webView.setWebViewClient(new MyWebViewClient()); progressbar=(ProgressBar)findViewById(R.id.progressBar); webView.setWebChromeClient(new WebChromeClient() { // this will be called on page loading progress @Override public void onProgressChanged(WebView view, int newProgress) { super.onProgressChanged(view, newProgress); progressbar.setProgress(newProgress); //loadingTitle.setProgress(newProgress); // hide the progress bar if the loading is complete if (newProgress == 100) { progressbar.setVisibility(View.GONE); } else{ progressbar.setVisibility(View.VISIBLE); } } }); } else { AlertDialog.Builder AD = new AlertDialog.Builder(this); AD.setIcon(R.drawable.ic_launcher); AD.setTitle("Not Connected To Wi-Fi/WLAN"); AD.setMessage("You need an Active Wi-Fi/WLAN on Alliance Broadband Network"); AD.setPositiveButton(android.R.string.ok, new OnClickListener() { @Override public void onClick(DialogInterface arg0, int arg1) { finish(); } }); AD.show(); } } public void otherWork(View promptsView) { AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder( this); // set prompts.xml to alertdialog builder alertDialogBuilder.setView(promptsView); final EditText userInput = (EditText) promptsView .findViewById(R.id.editTextDialogUserInput); // set dialog message alertDialogBuilder .setCancelable(false) .setPositiveButton("OK", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog,int id) { // get user input and set it to result // edit text //result.setText(userInput.getText()); SharedPreferences pos; String fileName = "file"; pos = getSharedPreferences(fileName, 0); SharedPreferences.Editor editor = pos.edit(); editor.putString("pwd", userInput.getText().toString()); editor.commit(); pos = getSharedPreferences(fileName, 0); String data = pos.getString("pwd", ""); URL = data.toString(); } }) .setNegativeButton("Cancel", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog,int id) { dialog.cancel(); } }); // create alert dialog AlertDialog alertDialog = alertDialogBuilder.create(); // show it alertDialog.show(); } @Override public boolean onKeyDown(int keyCode, KeyEvent event) { if(keyCode == KeyEvent.KEYCODE_BACK){ finish(); } return super.onKeyDown(keyCode, event); } private class MyWebViewClient extends WebViewClient { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { view.loadUrl(url); return true; } }<br>
LogCat
05-24 01:11:19.279: E/AndroidRuntime(3719): FATAL EXCEPTION: main 05-24 01:11:19.279: E/AndroidRuntime(3719): Process: com.mavenmaverick.ipconnect, PID: 3719 05-24 01:11:19.279: E/AndroidRuntime(3719): java.lang.RuntimeException: Unable to instantiate activity ComponentInfo{com.mavenmaverick.ipconnect/com.mavenmaverick.ipconnect.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.Object android.content.Context.getSystemService(java.lang.String)' on a null object reference 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2209) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2360) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.ActivityThread.access$800(ActivityThread.java:144) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1278) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.os.Handler.dispatchMessage(Handler.java:102) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.os.Looper.loop(Looper.java:135) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.ActivityThread.main(ActivityThread.java:5221) 05-24 01:11:19.279: E/AndroidRuntime(3719): at java.lang.reflect.Method.invoke(Native Method) 05-24 01:11:19.279: E/AndroidRuntime(3719): at java.lang.reflect.Method.invoke(Method.java:372) 05-24 01:11:19.279: E/AndroidRuntime(3719): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:899) 05-24 01:11:19.279: E/AndroidRuntime(3719): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:694) 05-24 01:11:19.279: E/AndroidRuntime(3719): Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.Object android.content.Context.getSystemService(java.lang.String)' on a null object reference 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.view.LayoutInflater.from(LayoutInflater.java:219) 05-24 01:11:19.279: E/AndroidRuntime(3719): at com.mavenmaverick.ipconnect.MainActivity.<init>(MainActivity.java:47) 05-24 01:11:19.279: E/AndroidRuntime(3719): at java.lang.reflect.Constructor.newInstance(Native Method) 05-24 01:11:19.279: E/AndroidRuntime(3719): at java.lang.Class.newInstance(Class.java:1572) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.Instrumentation.newActivity(Instrumentation.java:1065) 05-24 01:11:19.279: E/AndroidRuntime(3719): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2199) 05-24 01:11:19.279: E/AndroidRuntime(3719): ... 10 more
-
Admin almost 9 yearsCan you please elaborate a bit ?
LayoutInflater li = getLayoutInflater()
is not working ` -
CommonsWare almost 9 years@MavenMaverick: That is because you cannot call methods on the
Activity
superclass untilsuper.onCreate()
, except in certain situations, as I wrote in my answer. Please postpone your initialization ofli
until aftersuper.onCreate()
has been called. -
Admin almost 9 yearsYes! I fixed it. Now, on passing the input via AlertDialog, does not load the WebView. Any leads on that ?
-
Tash Pemhiwa almost 8 years@MavenMaverick if this solved your issue you should accept the answer