AttributeError: 'Tensor' object has no attribute 'numpy'
Solution 1
I suspect the place where you copied the code from had eager execution enabled, i.e. had invoked tf.enable_eager_execution()
at the start of the program.
You could do the same.
UPDATE: Note that eager execution is enabled by default in TensorFlow 2.0. So the answer above applies only to TensorFlow 1.x
Solution 2
Since the accepted answer did not solve the problem for me so I thought it might be helpful for some people who face the problem and that already have tensorflow version >= 2.2.0 and eager execution enabled.
The issue seems to be that for certain functions during the fitting model.fit()
the @tf.function
decorator prohibits the execution of functions like tensor.numpy()
for performance reasons.
The solution for me was to pass the flag run_eagerly=True
to the model.compile()
like this:
model.compile(..., run_eagerly=True)
Solution 3
Tensorflow 2 has a config option to run functions "eagerly" which will enable getting Tensor values via .numpy()
method. To enable eager execution, use following command:
tf.config.run_functions_eagerly(True)
Note that this is useful mainly for debugging.
See also: https://www.tensorflow.org/api_docs/python/tf/config/run_functions_eagerly
Solution 4
This can also happen in TF2.0 if your code is wrapped in a @tf.function or inside a Keras layer. Both of those run in graph mode. There's a lot of secretly broken code out of there because behavior differs between eager and graph modes and people are not aware that they're switching contexts, so be careful!
Solution 5
It happens in older version of TF. So try pip install tensorflow --upgrade
otherwise run
import tensorflow as tf
tf.enable_eager_execution()
If you are using Jupyter notebook, restart the Kernel.
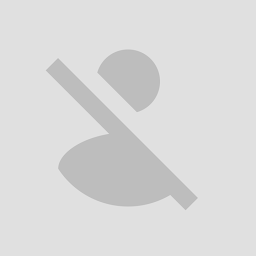
Frieder Hannenheim
Updated on July 15, 2022Comments
-
Frieder Hannenheim almost 2 years
How can I fix this error I downloaded this code from GitHub.
predicted_id = tf.multinomial(tf.exp(predictions), num_samples=1)[0][0].numpy()
throws the error
AttributeError: 'Tensor' object has no attribute 'numpy'
Please help me fix this!
I used:
sess = tf.Session() with sess.as_default(): predicted_id = tf.multinomial(tf.exp(predictions), num_samples=1)[0][0].eval()
And i get this error. Someone help me i just want it to work why is this so hard?
D:\Python>python TextGenOut.py File "TextGenOut.py", line 72 predicted_id = tf.multinomial(tf.exp(predictions), num_samples=1)[0][0].eval() ^ IndentationError: unexpected indent D:\Python>python TextGenOut.py 2018-09-16 21:50:57.008663: I T:\src\github\tensorflow\tensorflow\core\platform\cpu_feature_guard.cc:141] Your CPU supports instructions that this TensorFlow binary was not compiled to use: AVX2 2018-09-16 21:50:57.272973: W T:\src\github\tensorflow\tensorflow\core\framework\op_kernel.cc:1275] OP_REQUIRES failed at resource_variable_ops.cc:480 : Not found: Container localhost does not exist. (Could not find resource: localhost/model/embedding/embeddings) Traceback (most recent call last): File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 1278, in _do_call return fn(*args) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 1263, in _run_fn options, feed_dict, fetch_list, target_list, run_metadata) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 1350, in _call_tf_sessionrun run_metadata) tensorflow.python.framework.errors_impl.FailedPreconditionError: Error while reading resource variable model/dense/kernel from Container: localhost. This could mean that the variable was uninitialized. Not found: Container localhost does not exist. (Could not find resource: localhost/model/dense/kernel) [[Node: model/dense/MatMul/ReadVariableOp = ReadVariableOp[dtype=DT_FLOAT, _device="/job:localhost/replica:0/task:0/device:CPU:0"](model/dense/kernel)]] During handling of the above exception, another exception occurred: Traceback (most recent call last): File "TextGenOut.py", line 72, in <module> predicted_id = tf.multinomial(tf.exp(predictions), num_samples=1)[0][0].eval() File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\ops.py", line 680, in eval return _eval_using_default_session(self, feed_dict, self.graph, session) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\ops.py", line 4951, in _eval_using_default_session return session.run(tensors, feed_dict) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 877, in run run_metadata_ptr) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 1100, in _run feed_dict_tensor, options, run_metadata) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 1272, in _do_run run_metadata) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\client\session.py", line 1291, in _do_call raise type(e)(node_def, op, message) tensorflow.python.framework.errors_impl.FailedPreconditionError: Error while reading resource variable model/dense/kernel from Container: localhost. This could mean that the variable was uninitialized. Not found: Container localhost does not exist. (Could not find resource: localhost/model/dense/kernel) [[Node: model/dense/MatMul/ReadVariableOp = ReadVariableOp[dtype=DT_FLOAT, _device="/job:localhost/replica:0/task:0/device:CPU:0"](model/dense/kernel)]] Caused by op 'model/dense/MatMul/ReadVariableOp', defined at: File "TextGenOut.py", line 66, in <module> predictions, hidden = model(input_eval, hidden) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\keras\engine\base_layer.py", line 736, in __call__ outputs = self.call(inputs, *args, **kwargs) File "TextGenOut.py", line 39, in call x = self.fc(output) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\keras\engine\base_layer.py", line 736, in __call__ outputs = self.call(inputs, *args, **kwargs) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\keras\layers\core.py", line 943, in call outputs = gen_math_ops.mat_mul(inputs, self.kernel) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\ops\gen_math_ops.py", line 4750, in mat_mul name=name) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\op_def_library.py", line 510, in _apply_op_helper preferred_dtype=default_dtype) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\ops.py", line 1094, in internal_convert_to_tensor ret = conversion_func(value, dtype=dtype, name=name, as_ref=as_ref) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\ops\resource_variable_ops.py", line 1045, in _dense_var_to_tensor return var._dense_var_to_tensor(dtype=dtype, name=name, as_ref=as_ref) # pylint: disable=protected-access File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\ops\resource_variable_ops.py", line 1000, in _dense_var_to_tensor return self.value() File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\ops\resource_variable_ops.py", line 662, in value return self._read_variable_op() File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\ops\resource_variable_ops.py", line 745, in _read_variable_op self._dtype) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\ops\gen_resource_variable_ops.py", line 562, in read_variable_op "ReadVariableOp", resource=resource, dtype=dtype, name=name) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\op_def_library.py", line 787, in _apply_op_helper op_def=op_def) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\util\deprecation.py", line 454, in new_func return func(*args, **kwargs) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\ops.py", line 3155, in create_op op_def=op_def) File "C:\Users\fried\AppData\Roaming\Python\Python36\site-packages\tensorflow\python\framework\ops.py", line 1717, in __init__ self._traceback = tf_stack.extract_stack() FailedPreconditionError (see above for traceback): Error while reading resource variable model/dense/kernel from Container: localhost. This could mean that the variable was uninitialized. Not found: Container localhost does not exist. (Could not find resource: localhost/model/dense/kernel) [[Node: model/dense/MatMul/ReadVariableOp = ReadVariableOp[dtype=DT_FLOAT, _device="/job:localhost/replica:0/task:0/device:CPU:0"](model/dense/kernel)]]
-
Frieder Hannenheim over 5 yearsnow i get this error:raise ValueError("Cannot evaluate tensor using
eval()
: No default " ValueError: Cannot evaluate tensor usingeval()
: No default session is registered. Usewith sess.as_default()
or pass an explicit session toeval(session=sess)
-
Frieder Hannenheim over 5 yearsSorry if this is dumb but I'm very new to python.
-
Debosmit Ray over 5 yearsah - this is because you don't have a session established. updating the post above.
-
Debosmit Ray over 5 years@FriederMüller you should also use {this post} as reference
-
Frieder Hannenheim over 5 yearsThat's it thanks. What does eager execution do by the way?
-
ash over 5 yearsIt changes the TensirFlie APIs so that they execute operations on tensors immediately (as opposed to adding the operations to a graph). See the links in the answer above for details
-
Btc Sources over 4 yearsCould you please add a different reference in order to know how to do it without eager execution?
-
Will.Evo over 4 yearsThis is still a problem in TF 2.0.0
-
Helen over 4 yearsIncredible... thank you! I ran into the same while using an official TF tutorial :\
-
LKM about 4 yearsI got an error "ValueError: tf.enable_eager_execution must be called at program startup."
-
Smankusors about 4 yearsthe link is broken now, it says 404 page not found :(
-
Agustin Barrachina almost 4 yearsWell if you se the response the problem is that it should be eager execution. That's why is "fixed" in TF 2, because it has eager execution by default. But the problem is always the same, you cannot use
.numpy()
method in NOT eager execution. -
GuitarExtended almost 3 yearsPlease explain in what context you got this error.
-
jp_ over 2 yearshuh? it worked for me on tensorflow 2.0. ( not without eager execution)
-
BioCoder over 2 yearsThis solution works for me, it's perfect !
-
whitepanda over 2 yearsThis solves the problem for the latest version of tensorflow. +1
-
Admin about 2 yearsWhat's the workaround then? I need to use this in a tf.function
-
markemus about 2 years@Stark see Alexander's answer: stackoverflow.com/a/63596006/9095840 or perhaps stackoverflow.com/a/68057458/9095840
-
Admin about 2 yearsThanks for the reply. I did try that solution as well, but it did not work for me. I've found a way to implement what I wanted, without the tensor to numpy conversion however. I realised that I did not require it in the first place. So, it's all well.
-
Adri HM about 2 yearssolve my problem too. Thanks
-
ASLAN almost 2 yearsI think this is more up-to-date answer