Autodetect classes in Hibernate from jar
Solution 1
If I read you correctly, in the case where it does not work, the entities are in a different JAR than the persistence.xml that's used, right?
I think you're correct that's the problem. You need to tell Hibernate which JAR(s) to scan using the jar-file element. See the explanation and examples in the Hibernate docs here
Solution 2
I think Hibernate only scans for JPA Entities inside jar files, and not in classes/ folders for example, or it only scans in the jar with the persistence.xml, or something like that. Instead of trying to bend the Hibernate scanner, I felt it would be easier to scan for entities myself. It is a lot easier than I anticipated.
val entities = mutableListOf<Class<*>>()
AnnotationDetector(object : AnnotationDetector.TypeReporter {
override fun reportTypeAnnotation(annotation: Class<out Annotation>?, className: String?) {
entities.add(Class.forName(className))
}
override fun annotations(): Array<out Class<out Annotation>> = arrayOf(Entity::class.java)
}).detect("com.github")
VaadinOnKotlin.entityManagerFactory = Persistence.createEntityManagerFactory("sample", mapOf(AvailableSettings.LOADED_CLASSES to entities))
I have used the detector which is built-in in the atmosphere jar file; but you can use perhaps https://github.com/rmuller/infomas-asl or others as stated here: Scanning Java annotations at runtime The full code example is located here: https://github.com/mvysny/vaadin-on-kotlin/blob/master/vok-example-crud/src/test/java/com/github/vok/example/crud/Server.kt
None of the following helped: adding
<exclude-unlisted-classes>false</exclude-unlisted-classes>
to the persistence.xml
file did nothing (according to doc it is ignored in JavaSE anyway); adding
<property name="hibernate.archive.autodetection" value="class, hbm" />
to the persistence.xml
file did nothing (I'm not using hbm anyways). Don't waste your time trying to add those, they won't enable proper auto-scan.
Related videos on Youtube
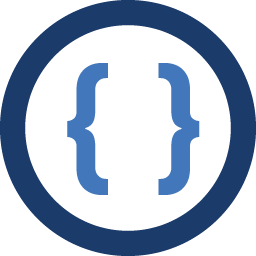
Admin
Updated on September 16, 2022Comments
-
Admin almost 2 years
I have a JavaEE project with several entities with the following
persistence.xml
file:... <properties> <!-- Scan for annotated classes --> <property name="hibernate.archive.autodetection" value="class"/> ...
And it seems to work. The project is then deployed as a JAR to two different projects. In one them, the
persistence.xml
file is basically the same as the one above, i.e. uses theautodetection
feature of Hibernate. However, it does not seem to work (because I think it needs to load search for the entities in a jar). When I tried manually listing all the entities in both xml files, everything works correctly.Edit: It works with jar-file but only with the absolute path to the jar. Pretty useless for a team project.
-
Admin almost 11 yearsBut what is the correct path for the jar file? Everything I try leads to a
The system cannot find the path specified
? -
Jeroen K almost 11 yearsThis stackoverflow question might help: what is the right path to refer a jar file in jpa persistence.xml in a web app?. Edit Oops, I just saw your edit - guess you figured it out already. Sorry, and yeah, I thought the same thing reading about the absolute path requirement.