Babel Preset does not provide support on IE11 for Object.assign - "Object doesn't support property or method 'assign'"
Solution 1
You need Babel Polyfill.
Either import it in your entry JS file, or use Webpack.
import "babel-polyfill";
or in webpack.config.js
module.exports = {
entry: ["babel-polyfill", "./app/main"]
}
NOTE : babel-polyfill
Should be imported on very top else It will not work
Solution 2
Babel Polyfill is outdated as of Babel 7.4.0
(Source)
Please use core-js instead.
import "core-js/stable";
import "regenerator-runtime/runtime";
Or with Webpack:
module.exports = {
entry: ["core-js/stable", "regenerator-runtime/runtime", "./app/main"]
}
In my case the above webpack config did not work! because I was also lacking a promise polyfill. This is the webpack.config I ended up using:
entry: { 'main': ['core-js/fn/promise', 'core-js/stable/object/assign', './wwwroot/src/app.js'] },
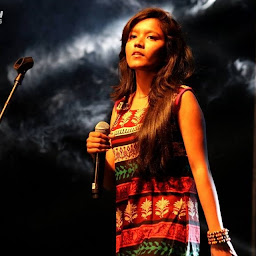
Ayesha Mundu
Updated on July 09, 2022Comments
-
Ayesha Mundu almost 2 years
I am using babel-preset-env version - 1.6.1 for my react app, i am getting a error on IE :- Object doesn't support property or method 'assign'
this is my .babelrc :-
{ "presets": [ "react", [ "env", { "targets": { "browsers": [ "last 1 versions", "ie >= 11" ] }, "debug": true, "modules": "commonjs" } ] ], "env": { "test": { "presets": [ [ "babel-preset-env", "react" ] ], "plugins": [ "transform-object-rest-spread", "transform-class-properties", "transform-runtime", "babel-plugin-dynamic-import-node", "array-includes", "url-search-params-polyfill", "transform-object-assign" ] } }, "plugins": [ "transform-object-rest-spread", "transform-class-properties", "syntax-dynamic-import", "transform-runtime", "array-includes", "url-search-params-polyfill", "transform-object-assign" ]
}
i tried these polyfills :-
https://babeljs.io/docs/plugins/transform-object-assign/ https://www.npmjs.com/package/babel-plugin-object-assign
but it didn't work
i am using syntax:-
let a = Object.assign({},obj);
everywhere in my project
i need a polyfill that would work for my project.