Back button on Windows Phone 8
Solution 1
You need to override OnBackKeyPress in your application's page and handle navigating the WebBrowser control back to the previous page.
Assuming you're using C#, here's roughly how you could do it (hook up WebBrowser_Navigated to your WebBrowser's Navigated event):
private Stack<Uri> _history = new Stack<Uri>();
private Uri _current = null;
protected override void OnBackKeyPress(CancelEventArgs e)
{
base.OnBackKeyPress(e);
if (_history.Count == 0)
{
// No history, allow the back button
// Or do whatever you need to do, like navigate the application page
return;
}
// Cancel the back button press
e.Cancel = true;
// Navigate to the last page
Browser.Navigate(_history.Peek());
}
private async void WebBrowser_Navigated(object sender, NavigationEventArgs e)
{
// If we navigated back, pop the last entry
if (_history.Count > 0 && _history.Peek() == e.Uri)
{
_history.Pop();
}
// Otherwise, if this isn't the first navigation, push the current
else if (_current != null)
{
_history.Push(_current);
}
// The current page is now the one we've navigated to
_current = e.Uri;
}
Solution 2
this worked with me, you can check if browser.CanGoBack() if true the go back else just return
protected override void OnBackKeyPress(CancelEventArgs e) {
base.OnBackKeyPress(e);
if (browser.CanGoBack)
{
e.Cancel = true;
browser.GoBack();
}
else {
return;
}
}
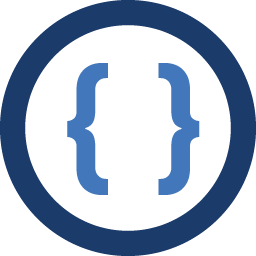
Admin
Updated on August 07, 2022Comments
-
Admin almost 2 years
I am having trouble getting the back hardware button to do what I would like it to do for the Windows Phone 8. The app is strictly just webview, so as of now when a back (hardware) button is clicked it closes the app. How can I work around this so it goes to the previous webpage or goes back to the index or something on those lines?
Thanks
This is what I currently have in the MainPage.xaml.cs file
namespace AvoidDiabetes { public partial class MainPage : PhoneApplicationPage { // Url of Home page private string MainUri = "/Html/index.html"; private Stack<Uri> _history = new Stack<Uri>(); private Uri _current = null; // Constructor public MainPage() { InitializeComponent(); } private void Browser_Loaded(object sender, RoutedEventArgs e) { // Add your URL here Browser.Navigate(new Uri(MainUri, UriKind.Relative)); Browser.IsScriptEnabled = true; } // Navigates back in the web browser's navigation stack, not the applications. private void BackApplicationBar_Click(object sender, EventArgs e) { Browser.GoBack(); } // Navigates forward in the web browser's navigation stack, not the applications. private void ForwardApplicationBar_Click(object sender, EventArgs e) { Browser.GoForward(); } // Navigates to the initial "home" page. private void HomeMenuItem_Click(object sender, EventArgs e) { Browser.Navigate(new Uri(MainUri, UriKind.Relative)); } // Handle navigation failures. private void Browser_NavigationFailed(object sender, System.Windows.Navigation.NavigationFailedEventArgs e) { MessageBox.Show("Navigation to this page failed, check your internet connection"); } protected override void OnBackKeyPress(CancelEventArgs e) { base.OnBackKeyPress(e); if (_history.Count == 0) { // No history, allow the back button // Or do whatever you need to do, like navigate the application page return; } // Otherwise, if this isn't the first navigation, push the current else { Browser.GoBack(); } } private async void WebBrowser_Navigated(object sender, NavigationEventArgs e) { // If we navigated back, pop the last entry if (_history.Count > 0 && _history.Peek() == e.Uri) { _history.Pop(); } // Otherwise, if this isn't the first navigation, push the current else if (_current != null) { _history.Push(_current); } // The current page is now the one we've navigated to _current = e.Uri; } }
}
-
Richard J. Ross III over 11 yearsAwesome. Ill try this in my monogame app tomorrow and get back to you!
-
Richard J. Ross III over 11 yearsThanks. Worked great. Have a bounty (in 4 hours), my pleasure :)
-
Admin over 11 yearsThanks so much, but I am sorry, but I am pretty new in programming and a little lost. When I added that into my web browswer's navigated page I was having trouble with the CancelEventArgs event being underlined as an error. Any suggestions?
-
Peter Huene over 11 yearsAre you "using System.ComponentModel;" in the source file?
-
Peter Huene over 11 yearsAt the top of the file you should see other using statements. If that one is missing, you needed to add it. It informs the compiler to search that namespace to resolve the CancelEventArgs type.
-
Admin over 11 yearsnot too sure how to add the code that I have into the comment to this, but I just added it up to the top in the original question. Any ideas what I should do from it?
-
Admin over 11 yearsokay I figured that part out, and I got the code to compile with no errors, but when I press the back button it is still closing the application. Maybe I added it at the wrong spot?
-
Peter Huene over 11 yearsSee the comment I added in OnBackKeyPress. It will currently back out of the page if there is no history (I.e. nothing to go back to in the browser). If you want it to do nothing, set e.Cancel to true there.
-
Admin over 11 yearshmm... yeah still not working for me, even when I navigate to many different pages, still gets out of the page. Thanks anyways
-
Admin over 11 yearsdamn, never mind, it's not working. It always thinks _history.Count = 0. It never registers more then that even when the user navigates from say index.html to 1.html
-
Peter Huene over 11 yearsIf you set a breakpoint on WebBrowser_Navigated, does it get hit (I assume you've hooked up the method to the Navigated event)?
-
Admin over 11 yearsI may be putting it in the wrong section, I have put the code that I have up top.
-
Peter Huene over 11 yearsAre you subscribing to the Navigated event in the XAML then? If not, you'll need to hook up the event after the InitializeComponent call.