Background sticky concurrent mark sweep GC freed
Solution 1
This is perfectly fine. It means that you're using memory, then its being freed by the GC. Its only a problem is either you go out of memory, or you see performance hiccups due to garbage collection. But its not anything you need to race to fix.
Solution 2
I have had the same issue. Your code will work, but if this hangs for too long, the app can get a App Not Responding error and close. It also defeats the purpose of using an AsyncTask.
The issue is that your AsyncTask contains a text view so it will block any other actions while executing. To fix this, make your AsyncTask static and pass it a reference to the Text view. Then store it in a WeakReference.
static class GetEmployee extends AsyncTask<Void,Void,String>{
WeakReference<TextView> textUserWeakReference;
WeakReference<TextView> textPassWeakReference;
GetEmployee(TextView textUser, TextView textPass) {
textUserWeakReference = new WeakReference<>(textUser);
textPassWeakReference = new WeakReference<>(textPass);
}
@Override
protected void onPreExecute() {
super.onPreExecute();
// loading = ProgressDialog.show(MainActivity.this,"Fetching...","Wait...",false,false);
}
@Override
protected void onPostExecute(String s) {
super.onPostExecute(s);
// loading.dismiss();
TextView textUser = textUserWeakReference.get();
TextView textPass = textPassWeakReference.get();
if(textView != null && textPass != null)
showEmployee(s, textUser, textPass);
}
@Override
protected String doInBackground(Void... params) {
RequestHandler rh = new RequestHandler();
String s = rh.sendGetRequestParam(DATA_URL,id);
return s;
}
}
private static void showEmployee(String json, TextView txtBoxUser, TextView txtBoxPassCode){
try {
JSONObject jsonObject = new JSONObject(json);
JSONArray result = jsonObject.getJSONArray(JSON_ARRAY);
JSONObject c = result.getJSONObject(0);
String name = c.getString(GET_BOXUSER);
String desg = c.getString(GET_PASSCODE);
txtBoxUser.setText(name);
txtBoxPasscode.setText(desg);
} catch (JSONException e) {
e.printStackTrace();
}
}
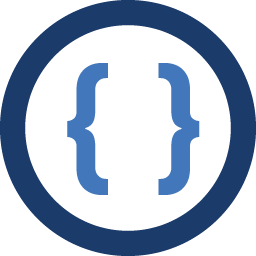
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have an application that checks the database every 10 seconds if there is any new data, if there is any data it will get it and stop checking the database.
I have implemented a text watcher to check if the textbox is empty. If it is, it will check the database, if it contains any text it will stop.
This is my code:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); txtBoxUser.addTextChangedListener(checkUserRent); getData(); } //TEXTWATCHER FOR GETTING PERSON WHO RENTED BOX private final TextWatcher checkUserRent = new TextWatcher() { public void beforeTextChanged(CharSequence s, int start, int count, int after) { } public void onTextChanged(CharSequence s, int start, int before, int count) { } public void afterTextChanged(Editable s) { if (s.length() == 0) { check(); } else { Toast.makeText(MainActivity.this, "STOP", Toast.LENGTH_LONG).show(); } } }; public void start(View view) { getData(); } public void cancel(View view) { txtBoxUser.setText(""); txtBoxPasscode.setText(""); } private void getData(){ final String id = txtBoxName.getText().toString().trim(); class GetEmployee extends AsyncTask<Void,Void,String>{ ProgressDialog loading; @Override protected void onPreExecute() { super.onPreExecute(); // loading = ProgressDialog.show(MainActivity.this,"Fetching...","Wait...",false,false); } @Override protected void onPostExecute(String s) { super.onPostExecute(s); // loading.dismiss(); showEmployee(s); } @Override protected String doInBackground(Void... params) { RequestHandler rh = new RequestHandler(); String s = rh.sendGetRequestParam(DATA_URL,id); return s; } } GetEmployee ge = new GetEmployee(); ge.execute(); } private void showEmployee(String json){ try { JSONObject jsonObject = new JSONObject(json); JSONArray result = jsonObject.getJSONArray(JSON_ARRAY); JSONObject c = result.getJSONObject(0); String name = c.getString(GET_BOXUSER); String desg = c.getString(GET_PASSCODE); txtBoxUser.setText(name); txtBoxPasscode.setText(desg); } catch (JSONException e) { e.printStackTrace(); } } private void check() { getData(); }
But I get this in the logcat while it is waiting for the data. I am just wondering is it okay or safe?
I/art: Background sticky concurrent mark sweep GC freed 5614(449KB) AllocSpace objects, 18(288KB) LOS objects, 33% free, 1691KB/2MB, paused 5.354ms total 10.731ms I/art: Background sticky concurrent mark sweep GC freed 7039(557KB) AllocSpace objects, 22(352KB) LOS objects, 39% free, 1561KB/2MB, paused 10.554ms total 15.931ms I/art: Background partial concurrent mark sweep GC freed 7279(564KB) AllocSpace objects, 21(336KB) LOS objects, 40% free, 1504KB/2MB, paused 5.721ms total 15.823ms I/art: WaitForGcToComplete blocked for 5.375ms for cause HeapTrim I/art: Background partial concurrent mark sweep GC freed 7650(591KB) AllocSpace objects, 22(352KB) LOS objects, 40% free, 1505KB/2MB, paused 5.511ms total 21.465ms