Bash script: Create a screen session and execute a command in it
Solution 1
You need you pass the command to run inside the screen session. You can do this by passing the command directly, or by calling a script which runs whatever command you wanted.
screen -S test "python3 test.py"
If you use this method, note that the command (python
) and the argument passed to it (test.py
) are both inside the speech marks " .. " - this can get complicated if you're passing arguments which require specificly formatted speech marks, and it maybe easier to then use the below method.
screen -S test bash -c "bash /home/user/test.sh"
where test.sh is in /home/user/, is executable (chmod +x ./test.py
) and contains:
#!/bin/sh
python3 test.py
The advantage of the second way is you can have as many commands as you want in the script, they'll run one after the other.
You can have multiple commands run 'at the same time' by using backgrounding of the task:
#!/bin/sh
# All 3 will run at the same time
python3 test.py &
python3 test1.py &
python3 test2.py &
Some extra clever tricks can be found at a similar question on SF, and one final thing to note is that with ALL these methods, the screen session itself will close once your program/script exits. If you don't want it to do this, you want to look at executing a bash session, which calls the script and then returns to the bash session after - like this screen -S test "someCommand; bash"
.
Solution 2
This might be achievable with -X argument. First you create a session and then you send the command to execute the script:
$ echo "echo Hello world > /tmp/foo" > /tmp/myscript.sh; chmod a+x /tmp/myscript.sh
$ screen -S mysession -d -m bash
$ screen -r mysession -X stuff "/tmp/myscript.sh"$(echo -ne '\015')
$ cat /tmp/foo
Hello world
The "stuff" command simulates pasting text to the terminal. The echo-command emulates hitting return. Good blog-post about sending commands: https://theterminallife.com/sending-commands-into-a-screen-session/
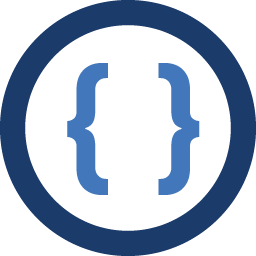
Admin
Updated on September 18, 2022Comments
-
Admin over 1 year
I want to write a bash file, which starts a screen session and executes a command inside.
The problem is, that it first creates a session and when you manually exited the session it executes the next command. Is there a way to execute the command inside of the session?
This is my code until now:
#!/bin/bash screen -S test python3 test.py
-
Josh over 3 yearsHaving the command as a string did not work for me instead this command did:
screen -S test python3 test.py
. You can usescreen -ls
to check -
djsmiley2kStaysInside over 3 years@Josh this is because your test.py while isn't setting the interpreter to python3, or because it's not executable. If you can run it directly (i.e.
./test.py
then it'll work. If not, you need to add the correct interpreter or permissions.