bash script loop multiple variables
11,466
Solution 1
To be generic, you can use 'length' as shown below.
#!/bin/bash
# Define the arrays
array1=("a" "b" "c" "d")
array2=("w" "x" "y" "z")
# get the length of the arrays
length=${#array1[@]}
# do the loop
for ((i=0;i<=$length;i++)); do
echo -e "${array1[$i]} : ${array2[$i]}"
done
You can also assign the array like the following
array1=`awk -F" " '$1 == "CLIENT" { print $2 }' clientserver.lst`
Solution 2
You can use arrays for that:
A=({a..z}) B=({1..26})
for (( I = 0; I < 26; ++I )); do
echo "/dev/sd${A[I]} /disk${B[I]} ext4 noatime 1 1" >> test
done
Example output:
/dev/sda /disk1 ext4 noatime 1 1
...
/dev/sdz /disk26 ext4 noatime 1 1
Update:
As suggested you could just use the index for values of B:
A=('' {a..z})
for (( I = 1; I <= 26; ++I )); do
echo "/dev/sd${A[I]} /disk${I} ext4 noatime 1 1" >> test
done
Also you could do some formatting with printf
to get a better output, and cleaner code:
A=('' {a..z})
for (( I = 1; I <= 26; ++I )); do
printf "%s%20s%15s%15s%4s%2s\n" "/dev/sd${A[I]}" "/disk${I}" ext4 noatime 1 1 >> test
done
Also, if you don't intend to append data to file, but only write once every generated set of lines, just make redirection by block instead:
A=('' {a..z})
for (( I = 1; I <= 26; ++I )); do
printf "%s%20s%15s%15s%4s%2s\n" "/dev/sd${A[I]}" "/disk${I}" ext4 noatime 1 1
done > test
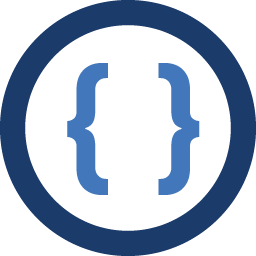
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to write something like following
for i in {a..z} && j in {1..26} do echo "/dev/sd"$i"1 /disk$j ext4 noatime 1 1" >> test done
Of course this is not correct syntax. Can someone please help me with correct syntax for doing this?
-
chepner over 10 yearsYou don't really need
B
, since${B[I]} == I
. -
konsolebox over 10 years@chepner Index starts from 0 actually but I can make an update based from that thanks.
-
chepner over 10 yearsOh, wait, I meant to say
${B[I]} == I+1
! Good catch :) -
Gallaecio over 8 yearsSince I am not allowed to perform a 1-character edit, please fix the for loop. You should use < instead of <=. Other than that, I find this answer the best one, thanks for your help!