bash script loop until webserver response 200
Solution 1
Don't reinvent the wheel. Plenty of tools do this already. Also, you check the status of the web server exactly once at the startup of the script (the curl
command doesn't get executed every time you reference the $HTTP variable, but only once when you initially define the variable. You would need to add the HTTP=\
curl` line into the loop.
Solution 2
Even in the edit you're checking for a condition in the outermost loop. Once that condition is satisfied that loop exits.
Your description sounds like you're trying to do
while true
do
HTTPD=`curl -A "Web Check" -sL --connect-timeout 3 -w "%{http_code}\n" "http://127.0.0.1" -o /dev/null`
until [ "$HTTPD" == "200" ]; do
printf '.'
sleep 3
service nginx restart
HTTPD=`curl -A "Web Check" -sL --connect-timeout 3 -w "%{http_code}\n" "http://127.0.0.1" -o /dev/null`
done
sleep 5
done
The outermost while true
is an infinite loop that will only exit when you kill the (checking) process
Related videos on Youtube
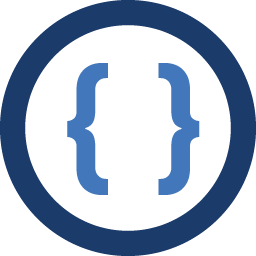
Admin
Updated on September 18, 2022Comments
-
Admin almost 2 years
I'm struggling to create a bash script that monitors the web service and if is down to restart the service in a while loop until it comes back up with a 200 status response.
Example :
#!/bin/bash HTTPD=`curl -A "Web Check" -sL --connect-timeout 3 -w "%{http_code}\n" "http://127.0.0.1" -o /dev/null` until [ "$HTTPD" == "200" ]; do printf '.' sleep 5 service nginx restart done or while [ "$HTTPD" != "200" ]; do service nginx restart sleep 5 if [ "$HTTPD" == "200" ];then break; else service nginx restart fi done
Both of them are working like 80% , the problem is that the loop will not re-check the status of $HTTPD response and break the loop.
I could add HTTPD=200; after restart line , but I want the script to check for the real status response.
EDIT: Working version
#!/bin/bash HTTPD=`curl -A "Web Check" -sL --connect-timeout 3 -w "%{http_code}\n" "http://127.0.0.1" -o /dev/null` until [ "$HTTPD" == "200" ]; do printf '.' sleep 3 service nginx restart HTTPD=`curl -A "Web Check" -sL --connect-timeout 3 -w "%{http_code}\n" "http://127.0.0.1" -o /dev/null` done