.BAT To .SH Converting?
The conversion to Bourne shell is pretty straightforward:
Add
#!/bin/sh
or similar as the first line, to tell Linux which interpreter to use. Unlike on Windows, there's more than one command shell, and more script interpreters than just shells besides.The equivalent of the
cmd.exe
commandCLS
isclear
on Linux.Linux is case-sensitive, so all your
ECHO
s have to be lowercase. If I'd kept yourIF
statements in the translated version, they'd have to be lowercase, too.-
echo
in shell scripts doesn't just print everything literally to the end of the line, as incmd.exe
. Bourne shell is a much richer language: it can apply meaning to what appears after a command before sending it to the command. In your code, the single quotes ('
) and multiple spaces won't survive this command processing.To avoid problems of this sort, I've double-quoted all of your
echo
strings. I could have done it selectively, double-quoting only the problem strings, but chose to double-quote everything for consistency. I don't want you to get the mistaken idea thatecho
in Bourne shell requires the double-quotes.If I wasn't interested in keeping the translation simple, so you can see more 1:1 correspondences between batch files and shell scripts, I'd replace the two big blocks of
echo
commands with a heredoc. echo.
is justecho
in Bourne shell. You don't need the dot in Bourne shell becauseecho
in Bourne shell isn't overloaded to turn command echoing on and off, as withECHO ON/OFF
incmd.exe
. (Bourne shell does have a similar feature, enabled viaset -x
.)It is possible to get colored output in Bourne shell, but there is no simple built-in command for it as in
cmd.exe
. If you want pretty colored menus, you can replace much of the code in this script with a call todialog(1)
.You use
read
to get input in a shell script;set
does other things.There is no
goto
in Bourne shell. We don't need it, because Bourne shell has decent control structures. I think acase
statement expresses the intent of your inner selection code, and an infinitewhile
loop expresses the outer "keep doing this until they hitq
" scheme.I don't see how code flow gets to your "press any key to continue" bit at the end, so I removed it. If I'm wrong, the rough equivalent of
PAUSE
isread -n 1 -s
.I have changed the calls to the external programs, dropping the
.exe
and changing.\
to./
to match the way things are done on Linux. You still need to come up with Linux equivalents ofVisualBoyAdvance.exe
anddesmume.exe
.
The result looks something like this:
#!/bin/sh
clear
while true do
echo "============= RawX GBA's ============="
echo "-------------------------------------"
echo "1. Pokemon Crystal"
echo "2. Pokemon Green"
echo "3. Pokemon Gold"
echo "4. Pokemon Pikachu"
echo "5. Pokemon Ruby"
echo "6. Pokemon Chaos Black"
echo "7. Pokemon Silver"
echo "8. Pokemon White (NDS)"
echo "9."
echo "10."
echo "11."
echo "12. Pokemon Black (NDS)"
echo "==========PRESS 'Q' TO QUIT=========="
echo
echo -n "Please select a number: "
read input
case $input in
1)
./VisualBoyAdvance "Pokemon Crystal.zip"
;;
2)
./VisualBoyAdvance "Pokemon Green.zip"
;;
# etc.
q)
clear
exit
*)
clear
echo "============INVALID INPUT============"
echo "-------------------------------------"
echo "Please select a number from the Main"
echo "Menu [1-12] or select 'Q' to quit."
echo "-------------------------------------"
echo "======PRESS ANY KEY TO CONTINUE======"
esac
done
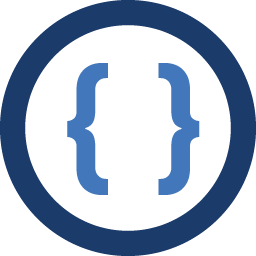
Admin
Updated on August 10, 2022Comments
-
Admin almost 2 years
Well I've written a bat file which is used to load my pokemon backups for when I'm away from my gameboys, however I've converted to linux and I'm having issues getting the .BAT file in my exe (I've decompiled the exe back the the source) to work as a .SH and I can't really find much on how to use shell commands as the same function they would be in a BAT file :/ I would also love to know how to set the SH file to load out of the current directory AND run said program in wine.
Here is my .BAT file which works 100% perfectly under windows but refuses to run under wine or a CMD prompt portable under wine
`:MENU CLS ECHO ============= RawX GBA's ============= ECHO ------------------------------------- ECHO 1. Pokemon Crystal ECHO 2. Pokemon Green ECHO 3. Pokemon Gold ECHO 4. Pokemon Pikachu ECHO 5. Pokemon Ruby ECHO 6. Pokemon Chaos Black Echo 7. Pokemon Silver ECHO 8. Pokemon White (NDS) ECHO 9. Echo 10. Echo 11. Echo 12. Pokemon Black (NDS) ECHO ==========PRESS 'Q' TO QUIT========== ECHO. color fc SET INPUT= SET /P INPUT=Please select a number: IF /I '%INPUT%'=='1' GOTO Selection1 IF /I '%INPUT%'=='2' GOTO Selection2 IF /I '%INPUT%'=='3' GOTO Selection3 IF /I '%INPUT%'=='4' GOTO Selection4 IF /I '%INPUT%'=='5' GOTO Selection5 IF /I '%INPUT%'=='6' GOTO Selection6 IF /I '%INPUT%'=='7' GOTO Selection7 IF /I '%INPUT%'=='8' GOTO Selection8 IF /I '%INPUT%'=='9' GOTO Selection9 IF /I '%INPUT%'=='10' GOTO Selection10 IF /I '%INPUT%'=='11' GOTO Selection11 IF /I '%INPUT%'=='12' GOTO Selection12 IF /I '%INPUT%'=='Q' GOTO Quit CLS ECHO ============INVALID INPUT============ ECHO ------------------------------------- ECHO Please select a number from the Main echo Menu [1-9] or select 'Q' to quit. ECHO ------------------------------------- ECHO ======PRESS ANY KEY TO CONTINUE====== PAUSE > NUL GOTO MENU :Selection1 :1 ".\VisualBoyAdvance.exe" ".\Pokemon Crystal.zip" goto menu :Selection2 :2 ".\VisualBoyAdvance.exe" ".\Pokemon Green.zip" goto menu :Selection3 ".\VisualBoyAdvance.exe" ".\Pokemon Gold.zip" goto menu :Selection4 ".\VisualBoyAdvance.exe" ".\Poke'mon Pikachu.zip" goto menu :Selection5 ".\VisualBoyAdvance.exe" ".\Pokemon Ruby.zip" goto menu :Selection6 ".\VisualBoyAdvance.exe" ".\Pokemon - Chaos Black.zip" goto menu :Selection7 ".\VisualBoyAdvance.exe" ".\Pokemon Silver.zip" goto menu :Selection8 ".\desmume.exe" ".\PokeWhite.zip" goto menu :Selection12 ".\desmume.exe" ".\PokeBlack.zip" goto menu :Quit CLS ECHO ==============THANK YOU=============== ECHO ------------------------------------- ECHO ======PRESS ANY KEY TO CONTINUE====== PAUSE>NUL EXIT`