BehaviorSubject adding the same value
995
What you're looking for is distinct()
method of BehaviorSubject()
.
Take a look at this from the documentation:
Skips data events if they are equal to the previous data event.
The returned stream provides the same events as this stream, except that it never provides two consecutive data events that are equal. That is, errors are passed through to the returned stream, and data events are passed through if they are distinct from the most recently emitted data event.
and here is how you implement it:
class TestBloc {
TestBloc() {
testBehavior.distinct((a, b) => a == b).listen((event) {
print('Event value = $event');
});
addValueToStream();
addValueToStream();
}
final testBehavior = BehaviorSubject<int>();
void addValueToStream() {
testBehavior.add(5);
}
}
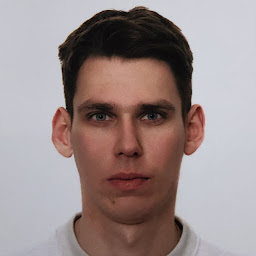
Author by
Anton Molchan
Updated on December 28, 2022Comments
-
Anton Molchan over 1 year
Hi I have a BehaviorSubject with a simple type int, I add the value 5 to it and then add another value 5. The stream listener sent me two events. How to force check the values and not send an event if the value is equal to the last value. Sample code:
class TestBloc { TestBloc(){ testBehavior.stream.listen((event) { print('Event value = $event'); }); addValueToStream(); addValueToStream(); } final testBehavior = BehaviorSubject<int>(); void addValueToStream() { testBehavior.add(5); } }
-
Anton Molchan about 3 yearsHi, @Stewie Griffin. For me so strange to need uses some additional function for streams for not send same values to stream. But it works fine, thank you. Maybe some need info how to use it in StreamBuilder:
StreamBuilder<int>( stream: bloc.myStream.distinct((a, b) => a == b), builder: (context, snapshot) {})
But more cool to use it in stream in bloc classfinal myBeh = BehaviorSubject<int>(); Stream<int> get myStream => myBeh.stream.distinct((a, b) => a == b);