belongs_to with :class_name option fails
39,985
Solution 1
class User < ActiveRecord::Base
has_many :books, :foreign_key => 'author_id'
end
class Book < ActiveRecord::Base
belongs_to :author, :class_name => 'User', :foreign_key => 'author_id', :validate => true
end
The best thing to do is to change your migration and change author_id
to user_id
. Then you can remove the :foreign_key
option.
Solution 2
It should be
belongs_to :user, :foreign_key => 'author_id'
if your foreign key is author id. Since you actually have User class, your Book must belong_to :user.
Solution 3
I do in this way:
Migration -
class AddAuthorToPosts < ActiveRecord::Migration
def change
add_reference :posts, :author, index: true
add_foreign_key :posts, :users, column: :author_id
end
end
class Post
belongs_to :author, class_name: "User"
Solution 4
migration
t.belongs_to :author, foreign_key: { to_table: :users }
working on rails 5
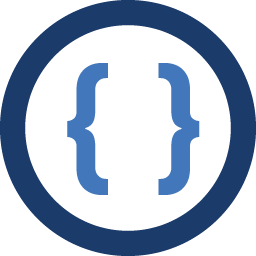
Author by
Admin
Updated on July 25, 2020Comments
-
Admin almost 4 years
I have no idea what went wrong but I can't get belongs_to work with :class_name option. Could somebody enlighten me. Thanks a lot!
Here is a snip from my code.
class CreateUsers < ActiveRecord::Migration def self.up create_table :users do |t| t.text :name end end def self.down drop_table :users end end ##################################################### class CreateBooks < ActiveRecord::Migration def self.up create_table :books do |t| t.text :title t.integer :author_id, :null => false end end def self.down drop_table :books end end ##################################################### class User < ActiveRecord::Base has_many: books end ##################################################### class Book < ActiveRecord::Base belongs_to :author, :class_name => 'User', :validate => true end ##################################################### class BooksController < ApplicationController def create user = User.new({:name => 'John Woo'}) user.save @failed_book = Book.new({:title => 'Failed!', :author => @user}) @failed_book.save # missing author_id @success_book = Book.new({:title => 'Nice day', :author_id => @user.id}) @success_book.save # no error! end end
environment:
ruby 1.9.1-p387 Rails 2.3.5