Best compiler warning level for C/C++ compilers?
Solution 1
This is a set of extra-paranoid flags I'm using for C++ code:
-g -O -Wall -Weffc++ -pedantic \
-pedantic-errors -Wextra -Waggregate-return -Wcast-align \
-Wcast-qual -Wchar-subscripts -Wcomment -Wconversion \
-Wdisabled-optimization \
-Werror -Wfloat-equal -Wformat -Wformat=2 \
-Wformat-nonliteral -Wformat-security \
-Wformat-y2k \
-Wimplicit -Wimport -Winit-self -Winline \
-Winvalid-pch \
-Wunsafe-loop-optimizations -Wlong-long -Wmissing-braces \
-Wmissing-field-initializers -Wmissing-format-attribute \
-Wmissing-include-dirs -Wmissing-noreturn \
-Wpacked -Wpadded -Wparentheses -Wpointer-arith \
-Wredundant-decls -Wreturn-type \
-Wsequence-point -Wshadow -Wsign-compare -Wstack-protector \
-Wstrict-aliasing -Wstrict-aliasing=2 -Wswitch -Wswitch-default \
-Wswitch-enum -Wtrigraphs -Wuninitialized \
-Wunknown-pragmas -Wunreachable-code -Wunused \
-Wunused-function -Wunused-label -Wunused-parameter \
-Wunused-value -Wunused-variable -Wvariadic-macros \
-Wvolatile-register-var -Wwrite-strings
That should give you something to get started. Depending on a project, you might need to tone it down in order to not see warning coming from third-party libraries (which are usually pretty careless about being warning free.) For example, Boost vector/matrix code will make g++ emit a lot of noise.
A better way to handle such cases is to write a wrapper around g++ that still uses warnings tuned up to max but allows one to suppress them from being seen for specific files/line numbers. I wrote such a tool long time ago and will release it once I have time to clean it up.
Solution 2
On Visual C++, I use /W4
and /WX
(treat warnings as errors).
VC also has /Wall
, but it's incompatible with the standard headers.
I choose to treat warnings as errors, because that forces me to fix them. I fix all warnings, even if that means adding #pragma
to ignore the warning - that way, I'm stating explicitly, that I'm aware of the warning (so other developers won't e-mail me about it).
Solution 3
I believe VC also supports
#pragma message ("note to self")
But as the system grows and grows, and you get a nightly build 30 developers work on simultaneously, it takes days to read all the notes to self, even in that amount that self is going to be do nothing but note reading and finally going to break under the stress not being able to keep up and have to resign...
No really, the amount of warnings is quickly going to grow if you allow them, and you won't be able to spot the really important ones (uninitialized variables, this pointer used in constructor, ...).
That's why I try to treat warnings as errors: most of the time, the compiler is right warning me, and if he isn't, I document it in the code and prepend
#pragma warning ( push )
#pragma warning ( 4191 : disable )
// violent code, properly documented
#pragma warning ( pop )
I just read they have a warning ( N : suppress )
pragma, too.
Solution 4
I tend to use -Wall
(because everyone does make bugs, nobody is perfect) , but i don't use -Werror
(treat warnings as errors) because now and then gcc warns about things which are right anyway (false positives).
Solution 5
I agree with litb to always use -Wall. In addition, if you want to ensure your code is compliant you can also use -pedantic. Another warning that can be helpful if you're handling unions and structs at the byte level is -Wpadded.
Related videos on Youtube
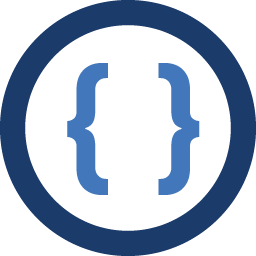
Admin
Updated on March 10, 2020Comments
-
Admin about 4 years
What compiler warning level do you recommend for different C/C++ compilers?
gcc and g++ will let you get away with a lot on the default level. I find the best warning level for me is '-Wall'. And I always try to remove fix the code for the warnings it generates. (Even the silly ones about using parenthesis for logical precedence rules or to say I really mean 'if (x = y)')
What are your favorite levels for the different compilers, such as Sun CC, aCC (HPUX ?), Visual Studio, intel?
Edit:
I just wanted to point out that I don't use "-Werror" (but I do understand it's utility) on gcc/g++ because, I use:
#warning "this is a note to myself"
in a few places in my code. Do all the compilers understand the #warning macro?
-
Mikeage over 15 years#warning is non-standard; I think #error is pretty universal, though
-
-
Paulius over 15 yearsSo, if gcc throws 100 warnings at you, do you review them all every time you compile? Otherwise, I don't see how you're dealing with the real warnings buried in the pile of the false-positives...
-
Admin over 15 yearsI don't use Werror either. There are warnings (unused functions) coming from flex/bison generated source code that would be difficult to suppress.
-
codelogic over 15 yearsYou can work around the flex/bison warnings by using the techniques described here: wiki.services.openoffice.org/wiki/Writing_warning-free_code
-
codelogic over 15 yearsPaulius, I find it unlikely that every time you compile that you will get fresh sets of 100s of warnings, assuming of course that code is compiled fairly frequently. I can see it happening the first time though.
-
Johannes Schaub - litb over 15 yearsPaulius, if it throws 100 warnings at me, i'm going to fix those so i only have a few warnings left that are i don't have to worry about. using good libraries make it possible not having pages of warning messages. also, what codelogic says.i have regular "warning killing" phases where i kill them :)
-
Admin over 15 yearscodelogic, thanks for the link. It turns out there is only one warning I have left in flex. I use: %option noyywrap %option nounput to suppress warnings about these functions. The one I can't get rid of: Scanner.cc:1241: warning: comparison between signed and unsigned integer expressions
-
strager over 15 yearsI use
-W
(aka-Wextra
) in addition to-Wall
as it catches some extra warnings mainly due to typos and forgotten code paths (oops, forgot to return something!). It's really helpful in catching silly bugs at the compile stage. -
strager over 15 yearsBetter yet, see the GCC manual: gcc.gnu.org/onlinedocs/gcc-4.3.2/gcc/Warning-Options.html
-
Paulius over 15 yearslitb, so what about the warnings that you "don't have to worry about"? do you just leave them? I would assume that the compiler would show those warnings every time it compiles. Over time, the number of such warnings would, probably, increase... How do you find the real warnings then?
-
Paulius over 15 yearsContinued: And if you're not leaving those warnings, then I don't see any reason not use -Werror, because you're fixing them all anyway...
-
Johannes Schaub - litb over 15 yearsPaulius, it happens quite seldomly that i get a warning that i won't fix. but when that's the case, then will indeed just leave it. emacs is fine in coloring the warnings, so i won't miss others :)
-
Johannes Schaub - litb over 15 yearsanyway, i feel it's better to enable all warnings, than enable only a few but enable -Werror just because otherwise one wouldn't be able to compile without errors. but this seems to be a quite subjective matter :)
-
David Thornley over 15 yearsWhen teaching C a long time ago, the supplied library headers caused warnings. Sigh.
-
Johannes Schaub - litb over 15 yearsDavid, gcc has special ways to deal with that: gcc.gnu.org/onlinedocs/cpp/System-Headers.html
-
Jonathan Leffler over 15 yearsgcc -ansi is equivalent to 'gcc -std=c89'; if that's what you want, it is OK, but if you prefer C99, use '-std=c99'.
-
David Stone about 12 yearsSomeone asked a similar question as gcc-specific, and this post was referenced. I updated this answer to remove duplicate warnings (for instance, -Wformat=2 implies -Wformat-y2k). See: stackoverflow.com/a/9862800/852254
-
Admin over 9 yearsg++ won't build with
-Wimplicit
, is C/ObjC specific. -
Lii over 8 yearsThere seems to be much redundancy here. For example (at least on GCC)
-Wall
implies-Wunused
. Also, this answer would be much better is you explained the flags.