Best practices constructing an empty array
Solution 1
First, it's a slice not an array. Arrays and slices in Go are very different, arrays have a fixed size that is part of the type. I had trouble with this at first too :)
- Not really. Any if the three is correct, and any difference should be too small to worry about. In my own code I generally use whatever is easiest in a particular case.
- 0
- Nothing, until you need to add an item, then whatever it costs to allocate the storage needed.
Solution 2
What is the performance cost of using arrays with unspecified capacity?
There is certainly a cost when you start populating the slice. If you know how big the slice should grow, you can allocate capacity of the underlying array from the very begging as opposed to reallocating every time the underlying array fills up.
Here is a simple example with timing:
package main
import "fmt"
func main() {
limit := 500 * 1000 * 1000
mySlice := make([]int, 0, limit) //vs mySlice := make([]int, 0)
for i := 0; i < limit; i++ {
mySlice = append(mySlice, i)
}
fmt.Println(len(mySlice))
}
On my machine:
time go run my_file.go
With preallocation:
real 0m2.129s
user 0m2.073s
sys 0m1.357s
Without preallocation
real 0m7.673s
user 0m9.095s
sys 0m3.462s
Solution 3
Is there any difference between the 3 ways to make an empty array?
if empty array
means len(array)==0
, the answer is no, but actually only myArr3==nil
is true
.
What is the default capacity of an array when unspecified?
the default capacity will be same with the len you specify.
What is the performance cost of using arrays with unspecified capacity?
none
Related videos on Youtube
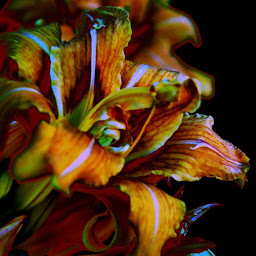
kpie
The ability to compute things beyond your minds capacity is one of the greatest privileges of the modern age.
Updated on September 16, 2022Comments
-
kpie almost 2 years
I'm wondering about best practices when initializing empty arrays.
i.e. Is there any difference here between arr1, arr2, and arr3?
myArr1 := []int{} myArr2 := make([]int,0) var myArr3 []int
I know that they make empty
[]int
but I wonder, is one syntax preferable to the others? Personally I find the first to be most readable but that's beside the point here. One key point of contention may be the array capacity, presumably the default capacity is the same between the three as it is unspecified. Is declaring arrays of unspecified capacity "bad"? I can assume it comes with some performance cost but how "bad" is it really?/tldr:
- Is there any difference between the 3 ways to make an empty array?
- What is the default capacity of an array when unspecified?
- What is the performance cost of using arrays with unspecified capacity?
-
kpie almost 7 yearsThanks for running the example!