Best way to create a maven artifact from existing jar
Solution 1
If you're not using a remote repository (which is a common situation for personal development), simply install
these artifacts in your local repository using the install:install-file
mojo:
mvn install:install-file
-Dfile=<path-to-file>
-DgroupId=<group-id>
-DartifactId=<artifact-id>
-Dversion=<version>
-Dpackaging=<packaging>
-DgeneratePom=true
Where: <path-to-file> the path to the file to load
<group-id> the group that the file should be registered under
<artifact-id> the artifact name for the file
<version> the version of the file
<packaging> the packaging of the file e.g. jar
But obviously, this will make your build non portable (this might not be an issue though). To not sacrifice the portability, you'll have to make the artifacts available in a remote repository. In a corporate context, the common way to deal with that is to install an enterprise repository (and in that case, to deploy
the artifacts indeed).
Update: Once your artifact is installed in your local repository, simply declare a <dependency>
element in your pom like for any other dependency, e.g.:
<dependency>
<groupId>aGroupId</groupId>
<artifactId>aArtifactId</artifactId>
<version>1.0.12a</version>
<packaging>jar</packaging>
</dependency>
Solution 2
You can use the Maven Deploy Plugin to upload your JAR file (and optionally a POM file, though by default one will be created for you) to your Maven repository.
Solution 3
As danben said, you'll have to deploy these jar to a repository. However, I seem to understand from your question that you don't have a repository except the global maven one.
You could use Nexus, or Artifactory.
Solution 4
I know your problem. Mavenizing jars is sometimes a pain (especially if they have further transitive dependencies, which also need to be defined in pom.xml).
Have you checked whether these libraries really never exist as maven deps? Have a look at the usual suspects:
Sometimes I like to use Nexus jar upload dialog to let create pom.xml files.
Solution 5
You can also create 'system' dependencies on jars that are not in a repository that are in the project. For example,
<dependency>
<groupId>com.example</groupId>
<artifactId>MySpecialLib</artifactId>
<version>1.2</version>
<scope>system</scope>
<systemPath>${basedir}/src/main/webapp/WEB-INF/lib/MySpecialLib-1.2.jar</systemPath>
</dependency>
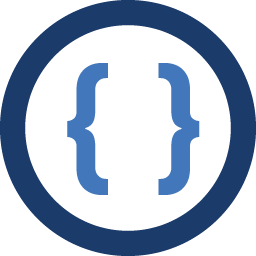
Admin
Updated on July 10, 2022Comments
-
Admin almost 2 years
I'm mavenizing some projects.
These projects all depend on a number of libraries, most of them are available in the maven repo. For the other libraries, I'd like to create a maven artifact, so I can use it as an dependency. The problem is, I only have jar files of these libraries.
What is the best way to create artifacts from existing jar files?