Binary Write using ASP.NET into a new tab / window
Solution 1
First, create an additional page named "PDF.aspx" or whatever you would like it called.
Second, in your page, store your "binarydata" variable in a session.
Session("binaryData") = binarydata
And tell it to go to your new page.
Response.Redirect("PDF.aspx")
Third, go to your PDF.aspx code behind page and put the following:
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Try
Response.ContentType = "Application/pdf"
Response.BinaryWrite(Session("binaryData"))
Response.End()
Catch ex As Exception
Throw ex
End Try
End Sub
That should produce the result you want. :)
Solution 2
I know this is old but here's what I had done to achieve the OP was after. (Forgive me as this is C# but it shouldn't be too hard to convert it)
This is the original code that I had used that displayed the same behavior that you have described!
Response.ClearContent();
Response.ContentType = "application/pdf";
Response.AddHeader("Content-Disposition", "inline; filename=" + docName);
Response.AddHeader("Content-Length", byteArray.Length.ToString());
Response.BinaryWrite(byteArray);
Response.End();
There are two key changes that I had to make to get this to work the way I wanted (Download the file as PDF, then open in a new tab when the user had clicked on that attachment.) and they are both on line 3 of the code above.
Change this
Response.AddHeader("Content-Disposition", "inline; filename=" + docName);
To this
Response.AddHeader("Content-Disposition", "attachment; filename=" + docName + ".pdf");
If you specify the Content-Disposition as "Inline" it will output the file content in your current window.
You need to change that disposition to "attachment" AND make sure your extension suffix is explicitly set to .pdf.
Solution 3
How about an alternative? Write to the browser some client side script to open a new window/tab.
ClientScript.RegisterStartupScript(this.GetType(), "scriptkey", "window.open('MyPDFDisplayer.ashx');");
Then create a new generic handler MyPDFDisplayer.ashx that writes the PDF to the window.
context.Response.ContentType = "application/pdf";
context.Response.BinaryWrite(binaryData);
context.Response.End();
I'm a C# guy, so you'll have to translate but it should be pretty easy.
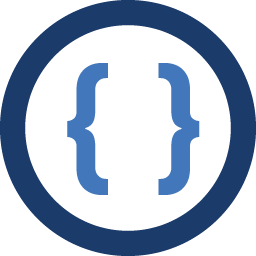
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I am using VB.NET to write a pdf file directly to the browser, which opens it right away replacing the content in a current window. I am trying to do is set a parameter which will cause binarywrite in a new tab / window.
' Set the appropriate ContentType. Response.ContentType = "Application/pdf" ' Write file directly to browser. Response.BinaryWrite(binaryData) Response.End()
There is has to be something that I can set which will cause this to write binary PDF in a new window. Like Response.Target = "_blank" ?????
-
Admin almost 10 yearsI thought there would be an easier way to do this but I guess that is the only way. Thank you.
-
mason almost 10 years@MikeR. PDF.aspx should likely be a generic handler (PDF.ashx), as you don't need the overhead of an entire page.
-
mason almost 10 years@WyattTroutman No problem. Generic handlers are very useful for obtaining documents or images or other binary files from a database. You can even use them to serve static (regular files) and use them for authorization or download statistics etc. One of the most common uses I see of generic handlers is retrieving images from a database for display in a GridView, with the image id being passed via query string.