Bitwise shift operators. Signed and unsigned
Solution 1
">>" is signed because it keeps the sign. It uses the most left digit in binary representation of a number as a filler. For example:
| this value is used as a filler
11011011
>> 11101101
01010010
>> 00101001
">>>" is unsigned version of this operator. It always use zero as a filler:
11011011
>>> 01101101
01010010
>>> 00101001
In binary representation the most left digit determines sign of the number. So, if it's '1' then we have negative value and if it's '0' - then our number is positive. That's why using the most left digit as a filler allows to keep sign permanent.
Solution 2
The idea behind the shifts is that they can act as multiplying and dividing by powers of 2 ( << 1 is equivalent to *= 2, >> 2 is equivalent to /= 4), which is why the signed version of shifting exists. Unsigned shifting doesn't preserve the sign, necessarily, though. The << operator doesn't actually preserve the sign, as you suggest; it simply happens to in your example. Try doing a left shift on 2,147,483,647; it doesn't stay positive. The reason that they don't bother trying to make a 'signed' left shift is because, if the number shifts from positive to negative (or viceversa), then you went outside the bounds of the variable type anyway.
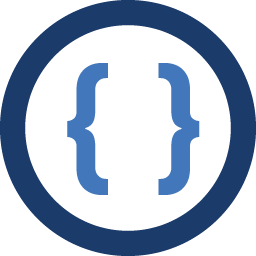
Admin
Updated on September 19, 2021Comments
-
Admin over 2 years
I'm practising for the SCJP exam using cram notes from the Internet.
According to my notes the
>>
operator is supposed to be signed right shift, with the sign bit being brought in from the left. While the left shift operator<<
is supposed to preserve the sign bit.Playing around however, I'm able to shift the sign with the
<<
operator (f.e.Integer.MAX_VALUE << 1
evaluates to-2
, while I'm never able to shift the sign with the>>
operator.I must be misunderstanding something here, but what?
-
Admin about 14 yearsAh, ok then I understand how the right shift operators are supposed to work. Thanks! But why am I able to change sign with "<<"?
-
Roman about 14 yearsBecause it shifts in another direction and the most left digit depends on the original number. It uses 0 as a filler but appends it to the right side of your number.
-
Stephen C about 14 yearsThe sign of an integer is determined by the value of its leftmost bit. If you shift in a
0
when it is1
or vice versa, the sign of the result changes. -
Admin about 14 yearsOk, so then basically the info in my notes is not correct on how the left shift operator (<<) works. If I get you right it: 1) Fills with 0s from the right. 2) WILL shift the (leftmost) sign bit and replace it with a bit from right.