BloC testing "The method xxx isn't defined for the type 'Object'" error
150
The method 'X' isn't defined for the type 'Object'.
error is usually thrown when the type of a specific object is not clear, hence it falls back to the general type Object
.
Based on the docs you provided, you should define the Cubit class type and it's state type inside blocTest
method:
blocTest<CounterCubit, CounterState>( // <-- Notice the defined types
"The cubit should emit a CounterState(countervalue:1, wasIncremented:true) when cubit.increment() is called.",
build: () => CounterCubit(), // <-- Create an instance of a Cubit
act: (cubit) => cubit.increment(), // <-- ! is not needed here since the type is defined
expect: () => [ // <-- "expect" should be defined as an array of expected states
CounterState(counterValue: 1, wasIncremented: true),
]
);
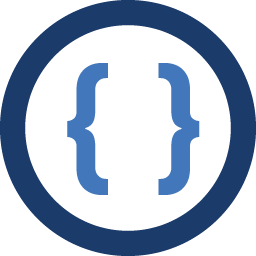
Author by
Admin
Updated on December 01, 2022Comments
-
Admin over 1 year
I am trying to learn testing with bloc. Followed the procedure as stated in docs.
I have lib/cubit/counter_cubit.dart and lib/cubit/counterState.dart files
counter_cubit.dart is:
import 'package:bloc/bloc.dart'; import 'package:equatable/equatable.dart'; part 'counter_state.dart'; class CounterCubit extends Cubit<CounterState> { CounterCubit() : super(CounterState(counterValue: 0, wasIncremented: false)); void increment() => emit( CounterState(counterValue: state.counterValue + 1, wasIncremented: true)); void decrement() => emit(CounterState( counterValue: state.counterValue - 1, wasIncremented: false)); }
counter_state.dart is:
part of 'counter_cubit.dart'; class CounterState extends Equatable { int counterValue; bool wasIncremented; CounterState({ required this.counterValue, required this.wasIncremented, }); @override List<Object?> get props => [counterValue, wasIncremented]; }
and the counter_cubit_test.dart is:
import 'package:bloc_basics/cubit/counter_cubit.dart'; import 'package:bloc_test/bloc_test.dart'; import 'package:flutter_test/flutter_test.dart'; void main() { group("CounterCubit", () { final CounterCubit counterCubit = CounterCubit(); test( "the initial state for the CounterCubitis CounterState(counterValue:0, wasIncremented:false)", () { expect(counterCubit.state, CounterState(counterValue: 0, wasIncremented: false)); }); blocTest( "The cubit should emit a CounterState(countervalue:1, wasIncremented:true) when cubit.increment() is called.", build: () => counterCubit, act: (cubit) => cubit!.increment(), expect: () => CounterState(counterValue: 1, wasIncremented: true)); }); }
That line
act: (cubit) => cubit!.increment()
incounter_cubit_test.dart
is throwingThe method 'increment' isn't defined for the type 'Object'.
Error.Couldn't figure out.
-
Admin about 2 yearsThaks a lot. It worked