Blur behind transparent WPF window
Solution 1
For anyone interested I have found a solution for Windows 10, It looks as though it isn't possible on Windows 8, like David Heffernan mentioned, DwmEnableBlurBehindWindow was removed from Windows 8, however Microsoft re-introduced a solution for achieving this effect in Windows 10.
- Windows 10: Solution using SetWindowCompositionAttribute
- Windows 8: No solution
- Windows 7: you can continue to use DwmEnableBlurBehindWindow
Solution 2
I hope I am not late to the party. You can use the SetWindowCompositionAttribute, but you are then forced to set the WindowStyle to "None" and implement your own native Window funtionality and handles. Also inheriting from a custom control is quite complicated. However, long story short. There's BlurryControls.
You can find it via NuGet by browsing for "BlurryControls" or check out the code yourself on GitHub. Eitherway, I hope this is helpful.
On GitHub you will also find a sample application.
Solution 3
Windows 8
As much as a dead end that'd better be completely forgotten, we might need to make sure our programs behave consistently across versions. If there is no need for a dynamic background and especially if the window is relatively small (a prime candidate would be a message box or similar over our own application window), the solution of capturing the screen behind the window and blurring it manually might work. In the Loaded
event handler:
var screen = window.Owner.PointToScreen(new System.Windows.Point((window.Owner.ActualWidth - window.ActualWidth) / 2, (window.Owner.ActualHeight - window.ActualHeight) / 2));
var rect = new Rectangle((int)screen.X, (int)screen.Y, (int)window.ActualWidth, (int)window.ActualHeight);
var bitmap = new Bitmap(rect.Width, rect.Height);
using (var graphics = Graphics.FromImage(bitmap))
graphics.CopyFromScreen(rect.Left, rect.Top, 0, 0, rect.Size);
border.Background = new ImageBrush(ApplyGaussianBlur(bitmap.ToBitmapImage())) {
TileMode = TileMode.None,
Stretch = Stretch.None,
AlignmentX = AlignmentX.Center,
AlignmentY = AlignmentY.Center,
};
where border
is an outermost Border
, normally left completely empty, only used on Windows 8.
The helper function is the usual bitmap conversion:
public static BitmapSource ToBitmapImage(this System.Drawing.Bitmap image) {
var hBitmap = image.GetHbitmap();
var bitmap = System.Windows.Interop.Imaging.CreateBitmapSourceFromHBitmap(hBitmap, IntPtr.Zero, Int32Rect.Empty, BitmapSizeOptions.FromEmptyOptions());
DeleteObject(hBitmap);
return bitmap;
}
ApplyGaussianBlur()
is best solved with a direct bitmap manipulation package like WriteableBitmapEx
, with the necessary modifications.
Related videos on Youtube
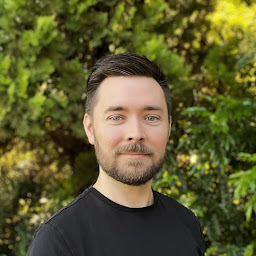
Tom
I am a polyglot software engineer that loves solving problems, I don't hold preference to any technology and I believe in choosing the right tools for the job, I really enjoy understanding complex solutions to complex problems and trying to see if they can be done in a more simplistic clean way.
Updated on September 14, 2022Comments
-
Tom almost 2 years
I am trying to create a WPF application with a semi transparent border-less window that blurs the background behind it.
Here is an example of what I want to do. Screenshot
I have tried to use
DwmEnableBlurBehindWindow
which works only on windows Vista/7.I am trying to find a solution that will work on Windows 7, 8 and 10.
-
David HeffernanThat's because MS removed the functionality, as I stated.
-
-
Tom almost 9 yearsI understand that the glass effect has been removed in windows 8, there must still be a way of achieving this effect, I have seen other programs do it for example Start10.
-
IInspectable almost 9 years@Tom: This doesn't look like a genuine Aero glass effect. From the looks it appears as though the application took a screenshot of the background and multiplied it onto its own background texture.
-
Tom almost 9 years@IInspectable: That's what I thought at first, however I ran a video behind it and you could see it moving, I tried the approach you mentioned, by taking a screenshot and adding it to the background and applying a blur effect, it does work, its just not ideal. and if the desktop changes while the window is open then the illusion is ruined.
-
IInspectable almost 9 years@Tom: I just installed Windows 10, and you are right: The Gaussian Blur is back for several UI elements (Start menu, clock/calendar, Action Center, etc.).
-
JazziJeff almost 7 yearsLink now dead :/ would be handy to place snippets of the code at the time as least SO stays around
-
Scott Solmer over 6 yearsLink to the Github Repo: github.com/riverar/sample-win10-aeroglass