Bootstrap button active and target styling not working
Solution 1
You should have a read here: https://developer.mozilla.org/en-US/docs/Web/CSS/Specificity
Basically you need to make sure that you give your styles a higher specificity than Bootstrap's styles, without using !important
.
The problem you're facing is that you use button.btn
instead of just .btn
. Since button
is a global selector and it has a lower specificity than a class, the Bootstrap's styles will still rule over your custom styles for the same elements. Even if your file is included after Bootstrap.
To fix this simply change your code to this:
.btn {
color: $white;
background-color: $lightOrange;
border-color: $darkOrange;
}
.btn:hover {
color: $white;
background-color: $mediumOrange;
border-color: $darkOrange;
}
.btn:active,
.btn:target {
color: $white;
background-color: $darkOrange;
border-color: $darkOrange;
}
Solution 2
When you're targeting bootstrap elements always keep in mind to follow and override all the possible combinations.
And in your case you're targeting bootstrap button
s, which are obviously having various combinations. Overriding them doesn't mean you've to use !important
its just you've to use right selector
in the right way.
As you've defined .btn:active
but a button
can be :active
as well as :focus
at the same point i.e. .btn:active:focus
. So if you want to override its :active
state then you've to look for all aspects as:
button.btn:active,
button.btn:focus,
button.btn:target,
button.btn:active:focus { ... /*write your CSS here */ }
Here I've created a JSFiddle also please have a look.
Hope it will help you solve your query and also clear your doubts regarding !important
.
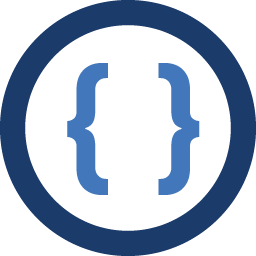
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I'm trying to customize my boostrap button colors, including on the hover state as well as when the user is busy clicking the button (button down) and after the user actually clicked the button. The sass that I have looks like this:
// Button styling button.btn { color: $white; background-color: $lightOrange; border-color: $darkOrange; } button.btn:hover { color: $white; background-color: $mediumOrange; border-color: $darkOrange; } button.btn:active, button.btn:target { color: $white; background-color: $darkOrange; border-color: $darkOrange; }
Now the default and hover states work great, but when the user clicks the mouse down on the button it defaults back to that gray color that is default with bootstrap. Also after the button has been clicked it remains that gray color instead of my
$darkOrange
that I specified. So in a nutshell, why doesn't:active
and:target
work? -
Gray Spectrum about 6 yearsThis was spot on for me. It was all about selector specificity. The funny thing is that Chrome's DevTools showed my CSS rule taking precedence, even though it wasn't. Once I made my selector more specific, this fixed the problem.