Button inside of anchor link works in Firefox but not in Internet Explorer?
Solution 1
You can't have a <button>
inside an <a>
element. As W3's content model description for the <a>
element states:
"there must be no interactive content descendant."
(a <button>
is considered interactive content)
To get the effect you're looking for, you can ditch the <a>
tags and add a simple event handler to each button which navigates the browser to the desired location, e.g.
<input type="button" value="stackoverflow.com" onClick="javascript:location.href = 'http://stackoverflow.com';" />
Please consider not doing this, however; there's a reason regular links work as they do:
- Users can instantly recognize links and understand that they navigate to other pages
- Search engines can identify them as links and follow them
- Screen readers can identify them as links and advise their users appropriately
You also add a completely unnecessary requirement to have JavaScript enabled just to perform a basic navigation; this is such a fundamental aspect of the web that I would consider such a dependency as unacceptable.
You can style your links, if desired, using a background image or background color, border and other techniques, so that they look like buttons, but under the covers, they should be ordinary links.
Solution 2
Just a note:
W3C has no problem with button inside of link tag, so it is just another MS sub-standard.
Answer:
Use surrogate button, unless you want to go for a full image.
Surrogate button can be put into tag (safer, if you use spans, not divs).
It can be styled to look like button, or anything else.
It is versatile - one piece of css code powers all instances - just define CSS once and from that point just copy and paste html instance wherever your code requires it.
Every button can have its own label - great for multi-lingual pages (easier that doing pictures for every language - I think) - also allows to propagate instances all over your script easier.
Adjusts its width to label length - also takes fixed width if it is how you want it.
IE7 is an exception to above - it must have width, or will make this button from edge to edge - alternatively to giving it width, you can float button left
- css for IE7:
a. .width:150px; (make note of dot before property, I usually target IE7 by adding such dot - remove dot and property will be read by all browsers)
b. text-align:center; - if you have fixed width, you have to have this to center text/label
c. cursor:pointer; - all IE must have this to show link pointer correctly - good browsers do not need it
You can go step forward with this code and use CSS3 to style it, instead of using images:
a. radius for round corners (limitation: IE will show them square)
b. gradient to make it "button like" (limitation: opera does not support gradients, so remember to set standard background colour for this browser)
c. use :hover pclass to change button states depending on mouse pointer position etc. - you can apply it to text label only, or whole button
CSS code below:
.button_surrogate span { margin:0; display:block; height:25px; text-align:center; cursor:pointer; .width:150px; background:url(left_button_edge.png) left top no-repeat; }
.button_surrogate span span { display:block; padding:0 14px; height:25px; background:url(right_button_edge.png) right top no-repeat; }
.button_surrogate span span span { display:block; overflow:hidden; padding:5px 0 0 0; background:url(button_connector.png) left top repeat-x; }
HTML code below (button instance):
<a href="#">
<span class="button_surrogate">
<span><span><span>YOUR_BUTTON_LABEL</span></span></span>
</span>
</a>
Solution 3
$("a > button").live('click', function() {
location.href = $(this).closest("a").attr("href");
}); // fixed
Solution 4
The code below will work just fine in all browsers:
<button onClick="location.href = 'http://www.google.com'">Go to Google</button>
Solution 5
You cannot have a button inside an a
tag. You can do some javascript to make it work however.
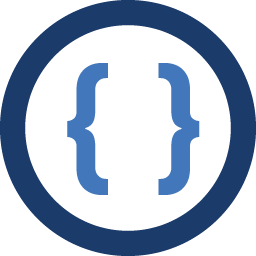
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Everything else in my site seems to be compatible with all browsers except for my links. They appear on the page, but they do not work. My code for the links are as follows-
<td bgcolor="#ffffff" height="370" valign="top" width="165"> <p> <a href="sc3.html"> <button style="width:120;height:25">Super Chem #3</button> </a> <a href="91hollywood.html"> <button style="width:120;height:25">91 Hollywood</button> </a> <a href="sbubba.html"> <button style="width:120;height:25">Super Bubba</button> </a> <a href="afgoohash.html"> <button style="width:120;height:25">Afgoo Hash</button> </a> <a href="superjack.html"> <button style="width:120;height:25">Super Jack</button> </a> <a href="sog.html"> <button style="width:120;height:25">Sugar OG</button> </a> <a href="91pk91.html"> <button style="width:120;height:25">91 x PK</button> </a> <a href="jedi1.html"> <button style="width:120;height:25">Jedi</button> </a> </p> <p> </p> <a href="http://indynile99.blogspot.com"> <button style="width:120;height:25">Blog</button> </a> <p> </p> </td>
-
Admin about 15 yearsWow, I caught most of that, a little bit over my head...so, if I understand correctly, either I use images for the buttons, or just turn them into ordinary links, right?
-
Will Eddins about 15 years@Jason Yes, usually you'd use an image for a link, or style the regular text link using CSS.
-
James McMahon over 14 yearsDoes the W3C dictate that you can't have a button in an anchor or is that just a Microsoft implementation?
-
James McMahon over 14 yearsThe problem with using images in lieu of buttons is that different interfaces are going to style buttons differently. So your button images may not match the rest of the interface. Anyone have a work around for this?
-
markus almost 13 yearsWhy can't you have button inside a? Other answers say you can, please link to rule or similar!
-
Cerin over 11 years"You can't have a <button> inside an <a> element." Says who? It works fine in nearly every browser, even IE9. Microsoft's incompetence no longer dictates web standards.
-
Eric over 11 yearsFor those that are wondering, this appears to be a bit of code that uses out of date jQuery functions. With current versions of jQuery you'll need to replace .live with .on. If you're using prototypejs instead, try starting with the $$ function.
-
Mike about 11 yearsThe HTML validator extension for firefox produces errors when I put a button in an anchor tag:
line 36 column 84 - Warning: inserting implicit <a> line 36 column 93 - Warning: discarding unexpected </button> line 36 column 53 - Warning: missing </button> line 36 column 37 - Warning: missing </a> before </div>
-
pkout about 11 yearsThis is not very good for search engine optimization, though. Not recommended if you want the page it links to get high ranking.
-
Odin over 10 yearsButton inside a link, neither link inside a button are not valid HTML. See another answer and explanation here: stackoverflow.com/questions/6393827/…
-
Odin over 10 yearsA button inside a link is not valid HTML and has undefined behavior.
-
Odin over 10 yearsFirst sentence is not true. <button> and <input> inside a link is not allowed in HTML spec.
-
James Donnelly over 10 years@Odin in HTML401 this is perfectly valid.
-
Odin over 10 yearsIt is valid only by omission. Nested link and button never had defined behavior. It's the same reasoning by which nested links are not allowed.
-
Ben Green over 10 yearsBad Microsoft implementation, also generally bad practice. There is nothing in the specification dictating buttons can't go inside links, but bad practice because you have 2 competing events which need to happen when a user clicks it - does it press the button or follow the link? It is this that confuses IE (along with a great many other things!) and a very good reason not to do it.
-
Dusty over 10 yearsI'm having problems with this in IE8
-
Kalmár Gábor over 10 yearsWhat is the version of jQuery you use? What is the exact problem?
-
dudewad almost 10 yearsI'd like to point out that the HTML markup here is hideous and an unacceptable solution to anyone who wants to write maintainable stuff. Having to remember this syntax once is difficult on its own, let alone any other hacks you might choose to employ. Rather, if you need to support IE 8, it might help to be okay with using markup that IE 8 supports. Call me old-hat. But I like code that works, not code that pretends to be something it's not.
-
dudewad almost 10 yearsIt's bad MSFT implementation. The spec for HTML5 states that the anchor tag can act as a block element.
-
Jeffz almost 10 yearsActually you should like it, because it works - which is down the line of your reasoning.
-
Ajeeb.K.P over 8 yearsDoes this answer the question?
Button inside of anchor link works in Firefox but not in Internet Explorer?
-
Jeff Puckett almost 8 yearsthis seemingly only works in Chrome, not in Firefox or IE
-
Jeff Puckett almost 8 yearsthis seemingly only works in Chrome, not in Firefox or IE
-
Jeff Puckett almost 8 yearsspecifically from the html5 button spec: "there must be no interactive content descendant." which lists the anchor tag as the first example.
-
Jeff Puckett almost 8 yearsspecifically from the html5 button spec: "there must be no interactive content descendant." which lists the anchor tag as the first example.
-
Christian Jensen almost 5 yearsAnswer has been updated with reference to W3's documentation.