c# bitmap filling
14,263
Solution 1
Just in case anyone needs a method solving this specific problem in a more general way, I wrote an extension method, taking colors and an integer that states how many tiles it should split off in x and y direction:
public static void FillImage(this Image img, int div, Color[] colors)
{
if (img == null) throw new ArgumentNullException();
if (div < 1) throw new ArgumentOutOfRangeException();
if (colors == null) throw new ArgumentNullException();
if (colors.Length < 1) throw new ArgumentException();
int xstep = img.Width / div;
int ystep = img.Height / div;
List<SolidBrush> brushes = new List<SolidBrush>();
foreach (Color color in colors)
brushes.Add(new SolidBrush(color));
using (Graphics g = Graphics.FromImage(img))
{
for (int x = 0; x < div; x++)
for (int y = 0; y < div; y++)
g.FillRectangle(brushes[(y * div + x) % colors.Length],
new Rectangle(x * xstep, y * ystep, xstep, ystep));
}
}
The four squares, the OP wanted would be produced with:
new Bitmap(160, 160).FillImage(2, new Color[]
{
Color.Red,
Color.Blue,
Color.Green,
Color.Yellow
});
Solution 2
You can try something like
using (Bitmap b = new Bitmap(160, 160))
using (Graphics g = Graphics.FromImage(b))
{
g.FillRectangle(new SolidBrush(Color.Blue), 0, 0, 79, 79);
g.FillRectangle(new SolidBrush(Color.Red), 79, 0, 159, 79);
g.FillRectangle(new SolidBrush(Color.Green), 0, 79, 79, 159);
g.FillRectangle(new SolidBrush(Color.Yellow), 79, 79, 159, 159);
b.Save(@"c:\test.bmp");
}
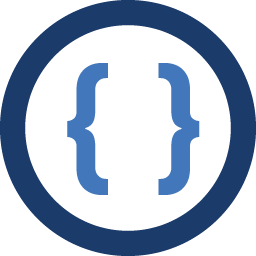
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to create a bitmap of size 160*160 and split it into four squares with each square filled with one color. How can this be done?
-
user1703401 about 14 yearsIt is off by one, Right and Bottom are not drawn. Just divide by 2.
-
Philip Daubmeier about 14 yearsThe fourth and fifth parameter of
FillRectangle
are width and height not 'end-x/y-values', so it should be 80 instead of 159. And like Hans Passant said, 80 instead of 79 as x and y parameters.